Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial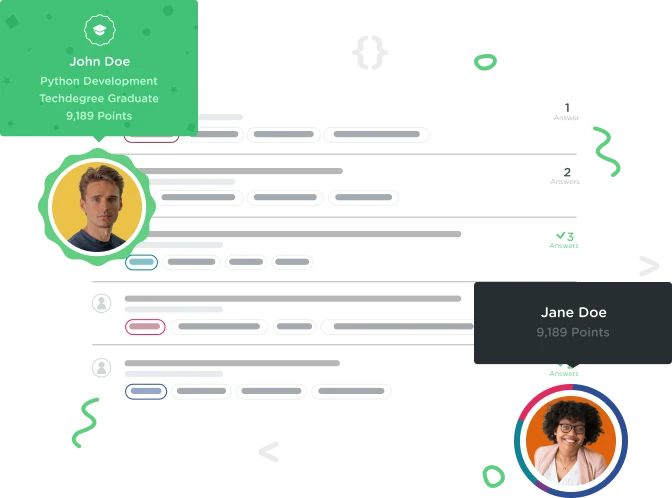
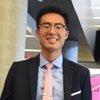
Paul Je
4,435 PointsNot sure why the protocol can't be the type of WifiLamp
Do the properties embedded in WIfiLamp and the protocol ColorSwitchable have to all be the same? I'm kind of confused and I don't really know what's going on for this chapter, I will see if I have to review some more of the videos again :( I thought I understood but I actually kind of don't. Why isn't the call statement at the end not working? This seems to make sense but I can't spot out why these two problems exist. Thanks for the help in advance !
protocol ColorSwitchable {
func switchColor(color: Color)
}
enum LightState {
case On, Off
}
enum Color {
case RGB(Double, Double, Double, Double)
case HSB(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .On
self.color = .RGB(0, 0, 0, 0)
}
}
var changedWifiLight = WifiLamp(state: LightState.On, color: Color.RGB(20.0, 30.0, 50.0, 1.0)
2 Answers
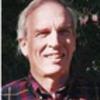
jcorum
71,830 PointsPaul, the function switchColor is written not to return anything, so it cannot have a return statement. If it returned a WiFiLamp it would be written this way:
func switchColor(color: Color) -> WifiLamp { . . . }
If you want to test your code in an Xcode playground you can use code like the following;
let wifi1 = WifiLamp() //create a WifiLamp object
print(wifi1.color) //display its color
print(wifi1.state) //display its state
let color1 = Color.RGB(235/255, 122/255, 14/255, 0.5) //create a Color object
wifi1.switchColor(color1) //call the switchColor() function
print(wifi1.color) //display the new color
You should get output like this:
RGB(0.0, 0.0, 0.0, 0.0)
On
RGB(0.92156862745098034, 0.47843137254901963, 0.054901960784313725, 0.5)
Here's all the code together in one place:
protocol ColorSwitchable {
func switchColor(color: Color)
}
enum LightState {
case On, Off
}
enum Color {
case RGB(Double, Double, Double, Double)
case HSB(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .On
self.color = .RGB(0,0,0,0)
}
func switchColor(color: Color) {
self.color = color
}
}
let wifi1 = WifiLamp() //create a WifiLamp object
print(wifi1.color) //display its color
print(wifi1.state) //display its state
let color1 = Color.RGB(235/255, 122/255, 14/255, 0.5) //create a Color object
wifi1.switchColor(color1) //call the switchColor() function with a Color object
print(wifi1.color) //display the new color
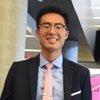
Paul Je
4,435 PointsThis is my code so far, but I can't implement it using your code - I'm not sure how to exactly set the new colour into the method to set the new colour to the lamp, any suggestions?
protocol ColorSwitchable { func switchColor(color: Color) }
enum LightState { case On, Off }
enum Color { case RGB(Double, Double, Double, Double) case HSB(Double, Double, Double, Double) }
class WifiLamp: ColorSwitchable { let state: LightState var color: Color
init(state: LightState, color: Color) {
self.state = .On
self.color = .RGB(0, 0, 0, 0)
}
func switchColor(color: Color) {
self.color = color
return WifiLamp(state: .On, color: .RGB(20.0, 30.0, 40.0, 1.0))
}
}
jcorum
71,830 Pointsjcorum
71,830 PointsPaul, to conform to the protocol you need to implement the method the protocol requires: