Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial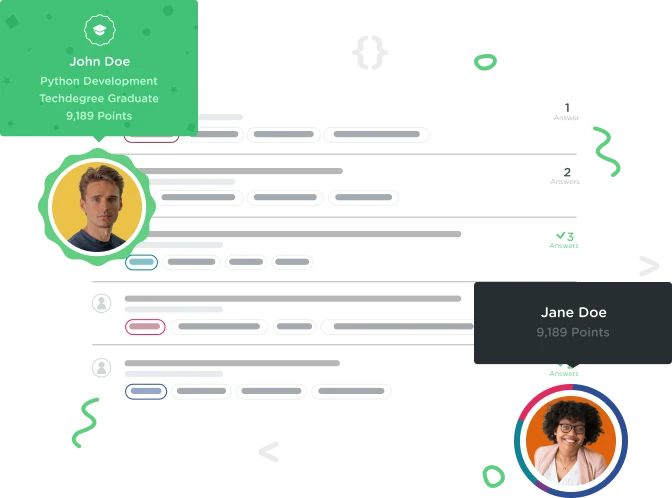
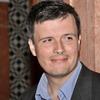
Brian Patterson
19,588 PointsNot sure why this is wrong?
Not sure why this code does not work in the final challenge? func isNotDivisable(dividend dividend: Int, divisor: Int) -> Bool? { if dividend % divisor != 0 { return true } else { return false } }
func isDivisible(#dividend: Int, #divisor: Int) -> Bool {
if dividend % divisor == 0 {
return true
} else {
return false
}
}
func isNotDivisable(#dividend: Int, #divisor: Int) -> Bool? {
if dividend % divisor != 0 {
return true
} else {
return false
}
}
6 Answers
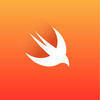
Steven Deutsch
21,046 PointsHey Brian,
Sorry I lost you, but the function is definitely not doing nothing!!! Sometimes it just looks as if its not doing anything, depending how we're viewing the output. I'm not sure what it is that you're trying to get to work, but take a look at this:
func isNotDivisable(dividend: Int, divisor: Int) -> String {
if dividend % divisor != 0 {
return "True"
} else {
return "Not true!"
}
}
let test = isNotDivisable(5, divisor: 2) // Will return the string "True"
Instead of returning the argument as a Bool, I changed the function's output to String. I then changed the function to return the strings "True" or "Not true!" based on how it evaluates.
func isNotDivisable(dividend: Int, divisor: Int) -> (Bool, String) {
if dividend % divisor != 0 {
return (true, "True")
} else {
return (false, "Not true!")
}
}
let test = isNotDivisable(5, divisor: 2) // Will return the Bool true and the string "True"
In this example I kept both the bool and the string output as a Tuple.
Hope this clarifies and doesn't make it worse!

sagearslan
2,276 PointsYou have a typo in the function name and unnecessary ? in return type.
Here's how it should be:
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
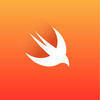
Steven Deutsch
21,046 PointsHey there Brian Patterson,
The question states "(Note: the function should return only a Bool and not an optional.)" Your function is currently trying to return an optional bool value.
This should fix it:
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
if dividend % divisor != 0 {
return true
} else {
return false
}
}
Good luck!
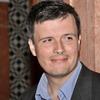
Brian Patterson
19,588 PointsThanks to everyone for the answer. But I do have one question when I call this function with the following why does it not say "Not True" if let finalResult = isNotDivisible(5, divisor: 2) { print("True") } else { print("Not true") }
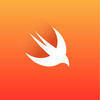
Steven Deutsch
21,046 PointsHey Brian,
That's because your isNotDivisible function is not evaluating any arguments that allow it to print "True" or "Not true".
Let me break down whats happening when you write:
let finalResult = isNotDivisible(dividend: 5, divisor: 2)
This takes the isNotDivisible function that you created above and passes it two parameters. The two parameters that it accepts are "dividend" and "divisor" which are of type Int. (You defined these parameter names and their type when creating your function.)
The function then uses these parameters to evaluate this argument defined in the function:
if dividend % divisor !=0 {
return true
} else {
return false
}
After evaluating the argument the function will return either true or false as a Boolean value based on what parameters were used. The function is evaluating to a Boolean value because you defined that output when creating your function.
func isNotDivisible(#dividend: Int, #divisor: Int) -> Bool {
}
Finally,
let finalResult = isNotDivisible(dividend: 5, divisor: 2)
After the function is evaluated, the Boolean value that is returned is set to the constant finalResult.
You have now created an instance of your isNotDivisible function named finalResult. Based on what you typed... It looks like you're trying to pass your function arguments outside of the function.
I hope I gave an accurate explanation as I'm still learning myself!
Good luck!
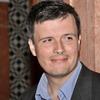
Brian Patterson
19,588 PointsYou kinda of lost me. How would you get the above argument to work? As I understand it the function is not really doing anything!!!
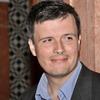
Brian Patterson
19,588 PointsThank you very much Steven for that it helps explains things better now. I think I was getting confused with the return value. If I needed it to say whether it was a true or not then I had to have a return value of a "String". This is the second time I have been through this course and it is slowly sinking in. I am very much looking forward to the updated videos as these are way over due!!! Again thanks for your patience for helping me out. Regards Brian