Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial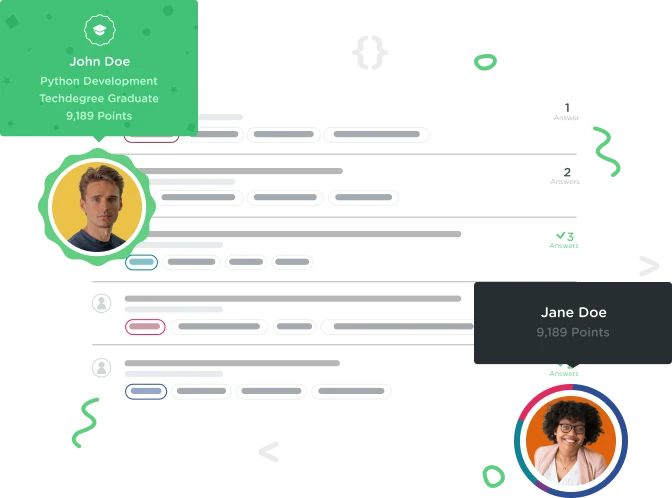
Matt Bentley
2,112 Pointsnot sure why this isn't compiling.
It compiles in xcode fine. version 6.4
class Button {
var width: Double
var height: Double
init(width: Double, height: Double){
self.width = width
self.height = height
}
class RoundButton: Button {
var cornerRadius = 5.0
}
}
var rounded = Button.RoundButton(width: 4.0, height: 2.0)
1 Answer
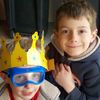
Chris Stromberg
Courses Plus Student 13,389 PointsYou have the class RoundButton included within your Button class check the use of your { }.
Your code should look like this....
class Button {
var width: Double
var height: Double
init(width: Double, height: Double){
self.width = width
self.height = height
}
}
You need to make the RoundButton class a subclass of Button, like I did below. Make sure the code below is not included in the original code block for the Button class.
class RoundButton: Button {
var cornerRadius = 5.0
}
Now put it all together....
class Button {
var width: Double
var height: Double
init(width: Double, height: Double){
self.width = width
self.height = height
}
}
class RoundButton: Button {
var cornerRadius = 5.0
}
Than you can do this. Create an instance of the RoundButton Class.
var rounded = RoundButton(width: 4.0, height: 2.0)