Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial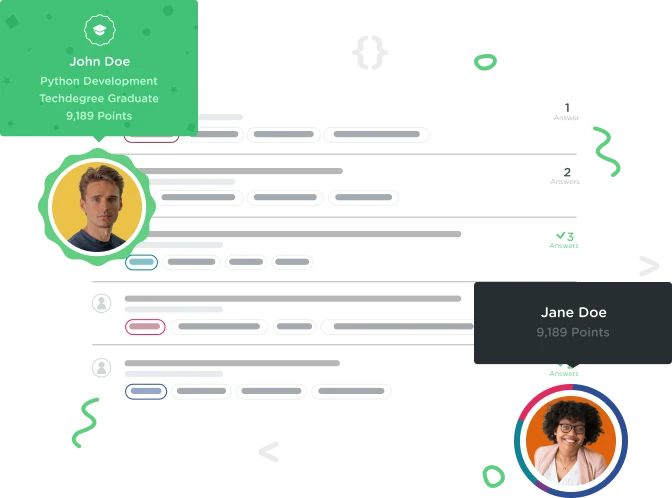

Keifer Joedicker
5,869 PointsNot sure why this isn't passing?
Did I misread the instruction or set the values wrong?
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self, width=3, height=3):
self.width = width
self.height = height
1 Answer
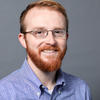
Kurt Maurer
16,725 PointsHey Keifer,
Thanks for the opportunity to review this stuff! I'm still working on developing my intuition with classes and inheritance, so if anyone else can elaborate, it would be much appreciated.
If we wanted a tictactoe board, we could do this:
TicTacToe = Board(3, 3)
However, the challenge wants us to make a subclass out of it, which means we want 3x3 to be the default values for the height and width. This will require overriding the init() of the Board() class. In your code, when you define the init(), you're essentially completely overriding the init() of the parent class. So your new TicTacToe() board would have height = 3, width = 3, but it wouldn't have a self.cells attribute. This is where super() comes in.
def __init__(self, width=3, height=3):
super().__init__(width, height)
This is saying "I want all of the attributes from the parent class(technically, the next thing on the method resolution order)." But then you can modify them, as we did here.
If you substitute this bit of code for your subclass, it will pass. Hope this helps!
Keifer Joedicker
5,869 PointsKeifer Joedicker
5,869 PointsThank you that makes a lot more sense!
Cristian Romero
11,911 PointsCristian Romero
11,911 PointsWow this is by far the best explanation I have come across! Thank you so much Kurt .