Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial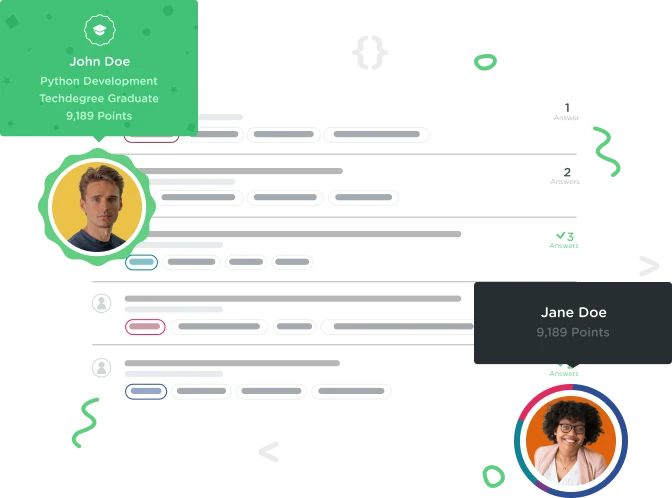

Logan Bresnahan
3,389 PointsNot totally understanding the special initialize method in Ruby. Do you have to use instance variables with initialize?
Can anyone break down the logic of initialize for me?
4 Answers

Ronald Jackson
9,229 PointsHi Logan -
To clarify a couple things regarding your undestanding about the initialize method:
First, when you create a new object (i.e., student) you must pass in the two arguments (name & grade). In my example, the initialize method expects to receive the two arguments. Once you associate an argument with the initialize method, you can not leave it off. If you do, it will generate an error.
Second, this leads to my second point of clarification. You wrote: "...every time I create a new instance of "class Student" that new instance will initialize whatever is defined by my initialize method." Not exactly .... The important thing to grasp is that it is the initialize method (not the new instance object) that receives the arguments you pass in. When you pass in the two arguments you're delivering them to the initialize method, so it can do its thing.
Nick Fuller expanded on my example by showing how you can call other methods from your initialize method. His expansion illustrates a very good point. Now, you asked what happens if you don't "add 'random_classroom' and 'assign_homework' [additional methods] within the initialize method?" The short answer is nothing. You don't need to add any additional methods unless your business needs require them. Nick was just illustrating a very good point about calling other methods with the initialize method.
By the way, I thought assigning homework on the first day was pretty funny ... seems like my John Smart will have a taskmaster for a teacher. lol
Hope it's clearer for you now.
Best,
Ronald

Nick Fuller
9,027 PointsHi Logan!
I think Ronald did a good job of answering your question, but I wanted to add 1 lil bit to it and that is you can call other methods from your initialize method. Let's build on the Student object that Ronald started.
class Student
def initialize(name, grade)
@name = name
@grade = grade
@classroom = random_classrom
assign_homework
end
def random_classroom
['12A', '15B', '16C', '18A'].sample
end
def assign_homework
@homework ||= Homework.last
end
end
Here we have set the students name and grade. But I'm also assigning the student to a random classroom (the sample method on the array class will randomly grab an element) and assigning them homework!
Does this make sense?

Logan Bresnahan
3,389 PointsOk, thank you, It seems simple enough that you could call another method from the initialize method, but let me ask you this. What would happen if you did not add "random_classroom" and "assign_homework" within the initialize method? Also, I apologize I'm not proficient in programming whatsoever.

Ronald Jackson
9,229 PointsFantastic Logan!
It's good to hear that the example and discussion helped you. It's also good that you did some independent reading on the subject. Treehouse courses are good, but it's important to research things from other sources too. I try to routinely look at as many different resources as I can lay my hands & eyes on (i.e., books, websites, tech forums, You-Tube videos, Coursera, etc.). After reviewing multiple sources, something that at first may have been cloudy, becomes clearer. Everyone has a different learning style, but that approach works best for me.
Here's a few tips that have helped me as I've traveled along the Rails Track. These strategies may also be of use to you.
First, try to learn as much Ruby as you can before tackling Rails. Some people may say it's not necessary to really grasp Ruby before tackling Rails, but I believe that having a decent handle on Ruby helps one to understand what's going on in Rails. Rails is like magic in many ways. It "hides" a lot of details behind the "curtain." It's great for getting stuff done, but it's possible to get something "done" in Rails without really understanding what you're doing. It's sort of like driving an automatic car without knowing how the transmission or engine works. So, I've tried my best to build up my understanding of Ruby to enhance my understanding of Rails. Hope that makes sense. My goal is to realy grasp what's going on under the Rails "hood." I think of Ruby as the key to unlock my Rails car (i.e., app).
Second, I've found that having a basic understanding of databases (MySQL) has helped me to better wrap my mind around Rails. Andew Chalkley's (Treehouse's) Database Foundations course is a good basic primer on the subject. After taking Andrew's course, I have a better understandng of and appreciation for the "magic", brilliance, and yes, beauty of ActiveRecord (the model) in Rails. The pieces began to make better sense.
Like you, Logan, I'm just learning new stuff and trying my best to understand it all. It's a bit overwhelming sometimes, but I try it step by step. A little each day adds up!
My last tip based on my experience so far: Be sure to give your brain proper rest! After I've rested, the fog almost always seem to lift. It's amazing how the human brain works! Whenever I get stuck (and that's often for me :-) I just look for new and different resources to enhance my understanding. Eventually it clicks.
Hope these tips are useful to you. Keep going! Good luck in your journey, wherever it may lead you.
Best,
Ronald

Nick Fuller
9,027 Points+1 props for this

Logan Bresnahan
3,389 PointsRonald and Nick,
You guys have been great! Ronald, thanks for the advice there. I would rather understand the building blocks of Ruby first. For whatever reason if I don't understand how the small parts work I never feel like I know what I'm doing! I realize I've got a long way to go but I love it. I find that all this keeps me very entertained because it's totally alien to me.
I'll definitely be absorbing as much info as I can and make sure I understand MySQL when I get to it. It's great to know there are people like you guys to help me out online and I'll pick your brains if I have any troubles in the future!
Thanks, Logan

Ronald Jackson
9,229 PointsHi Logan,
The initialize method allows you to pass arguments to a new object when you create it. For example, say you have the following
class Student
def initialize(name, grade)
@name = name
@grade = grade
end
Now say you create a new object (i.e., a real student). You "initialize" the object by passing in the arguments (i.e., name and grade):
student = Student.new ("John Smart", "First Grade")
Note that @name and @grade are instance variables, which are denoted by the @ sign. So, the Student class in my example presents an initialize method that takes a name and grade argument, and then "assigns" them to the two instance variables (ie. name and grade). The "instance variables" need to be "initialized" by passing in the two arguments to Student.new.
I hope this clarifies things for you.
Best of luck,
Ronald

Logan Bresnahan
3,389 PointsOk, I think I understand. If I were to put this in layman's terms, we are just using the initialize method so that every time I create a new instance of "class Student" like... "student" in the above example we have the option to add arguments that will be set to the instance variables within our initialize method. So, every time I create a new instance of "class Student" that new instance will initialize whatever is defined by my initialize method. Does that sound right?
Logan Bresnahan
3,389 PointsLogan Bresnahan
3,389 PointsYes, thank you, you guys have been great! I think I have a good grasp on the initialize method now. Thank you for also clarifying those points and examples above because I was off in my understanding. I did do a little light reading on objects and how Class is an object and how Object comes with predetermined methods associated with it written in Ruby, which in turn helped me understand why we were using the initialize method.
Thanks again! Logan