Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial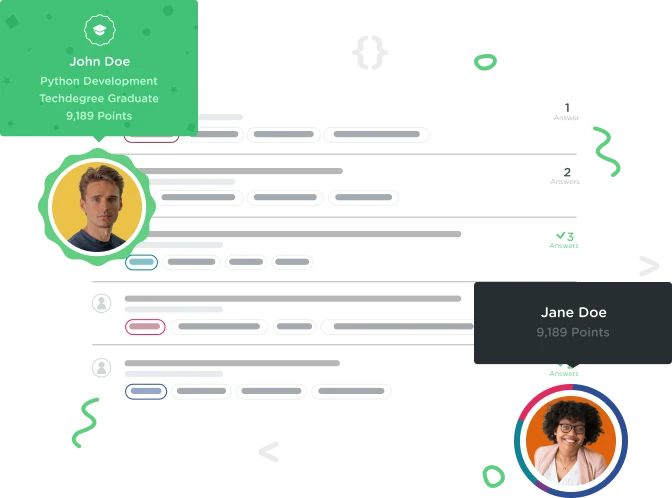

mustafa attaie
8,068 PointsNot understanding functions and arguements
I'm not sure if i just don't understand how this question is phrased or if I'm actually not understanding it? Can anyone give me some insight on what I'm doing wrong and mind clarifying exactly what I'm doing wrong?
function max(5,2) {
if(5 > 2) {
return 5
}
}
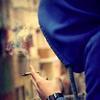
Tushar Singh
Courses Plus Student 8,692 Points// function name "max"
function max(num1, num2) { // arguments num1, num2( you can name them "a" and "b", or x, y, lemon, anything )
if (num1 > num2) {
return num1;
} else {
return num2;
}
};
alert(max(5,10)); // 2nd challenge
// you can use any numbers you want
max(6,10);
// just changed the arguments
So, basically a function is a set of instructions .In this case our function is set to give the max value every time an argument is passed. So now what are arguments? Well, arguments are nothing just you can say values which you want to use.
You just created a function "max" which takes two arguments and you can name it anything you want. What this function is doing basically it just returns the number which has the higher value than the other.
Added if else statements because what if the user types a higher value in the 1st argument and later on decides that may be for no reason the higher value looks nice in the second argument, then what? thats why an else statement.
I hope it helps ;)
1 Answer
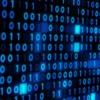
Alexander Davison
65,469 PointsYou are doing a wonderful job! There is still a couple problems, though. So first, you cannot have numbers as the first letter in a variable or argument name. Second, you need to add an else clause to the "if" condition. That's it for the first task. For the second task, you should alert the result of "max(<num1>, <num2>)". You can use any integer (number) to replace the <num1> and <num2>, but I choose 10 and 15. This is the full code:
function max(a, b) {
if (a > b) {
return a;
} else {
return b;
}
}
alert( max(10, 15) );
Hope that helps!
Shaheer Mir
11,599 PointsShaheer Mir
11,599 PointsHi, The function should work for any two numbers, not just 5 and 2. The two numbers that your function takes in are known as arguments. You can name these arguments whatever you like. The function should look something like the following:
Now, you can call the function using any two numbers, and the function will return the larger of the two numbers. e.g. max(5,2);