Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial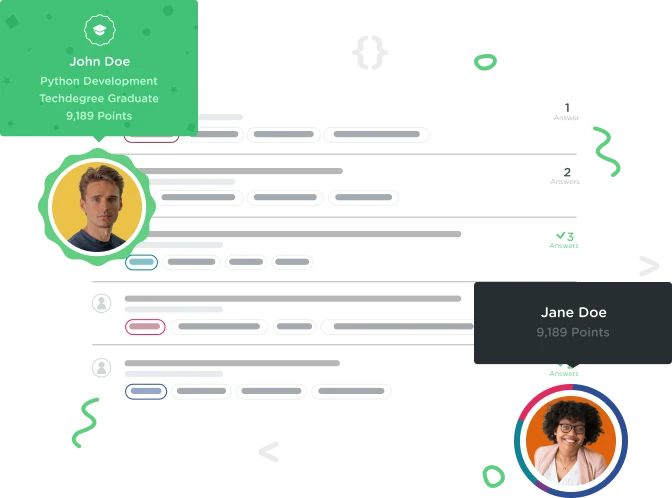

Hamzah Iqbal
Full Stack JavaScript Techdegree Student 11,145 PointsNot understanding passing in function parameters
Im not getting that when creating the function we have to define the parameter of the function. Then in the function we have to use:
function isValidUsername(username) {
return /[a-z]+/.test(username) //What is being passed into the username? Its not defined anywhere
3 Answers
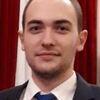
Victor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsI think this might help you. I only took the lines of code relevant to your question.
function isValidUsername(username) {
return /^[a-z]+$/.test(username);
}
function createListener(validator) {
return e => {
const text = e.target.value;
const valid = validator(text);
const showTip = text !== "" && !valid;
const tooltip = e.target.nextElementSibling;
showOrHideTip(showTip, tooltip);
};
}
usernameInput.addEventListener("input", createListener(isValidUsername));
This event listener created on the usernameInput
calls the function createListener
with isValidUsername
as an argument. In createListener
function, this argument is replaced by validator
parameter, so when you call validator(text)
you acctually call isValidUsername(text)
(text
here is the argument), where text
is actually what the user writes. Now look where isValidUsername
is declared. Replace the parameter username
with the argument text
, and there you go. username
is actually the text that the user inputs in the username field.
Hope this makes sense.
Maybe someone can reformulate this better.
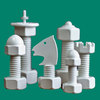
Steven Parker
230,274 PointsSee if this helps:
function isValidUsername(username) { // <-- the "username" parameter is defined here
Then, elsewhere in the program, this function will be called, perhaps something like this:
var isOK = isValidUsername(possibleName); // check what's in "possibleName" for validity
So in this case, when the function runs, whatever was in "possibleName" will now be in "username". This allows the code in the function to be written without knowing what the function will be used on later. Plus, it could get used more than once on different things, but it will still do the same job each time.

Hamzah Iqbal
Full Stack JavaScript Techdegree Student 11,145 PointsI get it. But the username gets passed in as a parameter. But it doesn’t even exist yet. I would understand with your example
var isOK = isValidUsername(possibleName) But nothing is being passed that is being tested so to say.
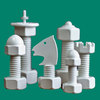
Steven Parker
230,274 PointsIn this example, the contents of "possibleName" is what is being passed. It would contain a string to be tested.
karan Badhwar
Web Development Techdegree Graduate 18,135 Pointskaran Badhwar
Web Development Techdegree Graduate 18,135 PointsHi Victor Stanciu, Thanks for the explanation, I helped, but I want to clarify again. So the function isValidUsername(username) gets called at this line right
const valid = validator(text);
and then returns a boolean value. Is my understanding of this right?