Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial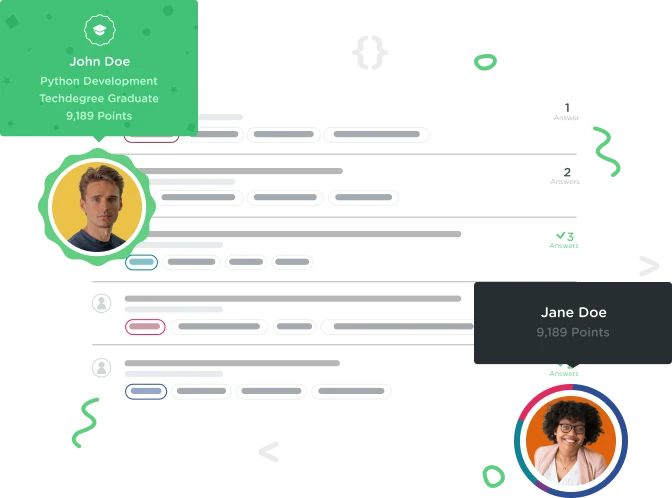

Hudson Lanier
6,329 PointsNot understanding this error message as it relates to kwargs
I'm getting an error message "couldn't find the RaceCar class." I'm not really sure why, as the RaceCar class is literally the first line of code. I don't understand why this doesn't work. I've made a "for" loop that will handle my kwargs, and I've assigned a key (laps) and value (0) to the RaceCar class. This should work, right?
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
RaceCar.color = color
RaceCar.fuel_remaining = fuel_remaining
RaceCar.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
RaceCar(laps=0)
def run_lap(length):
fuel_remaining += fuel_remaining - length*0.125
laps +=1
6 Answers

Hudson Lanier
6,329 PointsNevermind, got it! I had an indentation problem. Thanks Adam!

Simphiwe Masukume
7,030 PointsYou're required to set the atributes in the init method on the instance of the class not the class itself. Self represents an instance of a class, so they must be set on self and not the RaceCar class
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.laps = 0
Also, you're meant to subtract length*0.125 from fuel_remaining. Not add. Instead of += use -=
self.fuel_remaining -= length *0.125

Adam Kielar
Python Web Development Techdegree Graduate 13,818 PointsHello Hudson,
You have to instantiate color, fuel_remaining and laps, using "self" not RaceCar:
class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
Same problem is with run_lap class method:
def run_lap(self, length): self.length = length self.fuel_remaining -= (length * 0.125) self.laps += 1

Hudson Lanier
6,329 PointsThanks Adam, but it's still not working even after these adjustments. I'm really stumped here.
Python code
class RaceCar:
def __init__(self, color, fuel_remaining, laps=0, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.length = length
self.fuel_remaining -= (length*0.125)
self.laps += 1
```

Simphiwe Masukume
7,030 PointsThere is no need to return anything

Hudson Lanier
6,329 PointsI'm still not getting it to work

Simphiwe Masukume
7,030 Pointslets see what you have done

Hudson Lanier
6,329 Pointsclass RaceCar: def init(self, color, fuel_remaining, laps=0, **kwargs): self.color = color self.fuel_remaining = fuel_remaining self.laps = laps for key, value in kwargs.items(): setattr(self, key, value)
def run_lap(self, length):
return self.fuel_remaining -= length*0.125
self.laps+= 1