Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial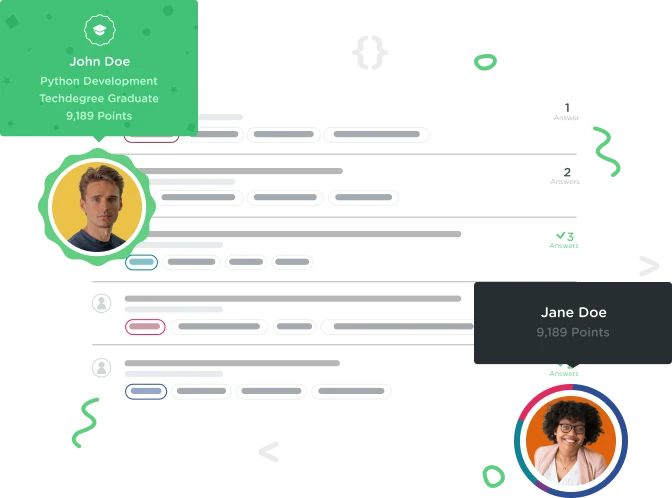
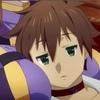
Edward A. Polanco Murillo
2,872 PointsNot understanding this particular code.
For some reason I am having difficulty with this particular piece of code. I cant seem to understand what I am doing wrong. I am I missing something?
Also anyone here who is doing Python or coding in general do you guys suffer from imposter syndrome & do you guys just stair at the computer screen and just feel lost and just feel like you will never understand how to do this or code for that matter, because for me I feel like I am screwing up and as a result I get frustrated (especially with OOP) and I just want to put down the keyboard and just do something else.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
@property
def __init__(self, *args, **kwargs):
super().init(size=2, die_class=D6, *args, *kwargs)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
1 Answer
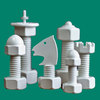
Steven Parker
230,274 PointsYou're pretty close, but:
- the "
__init__
" method is not a property - the super's method name is also "
__init__
" (not just "init
") - when expanding kwargs, use the double "splat" ("
**kwargs
" instead of "*kwargs
")