Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial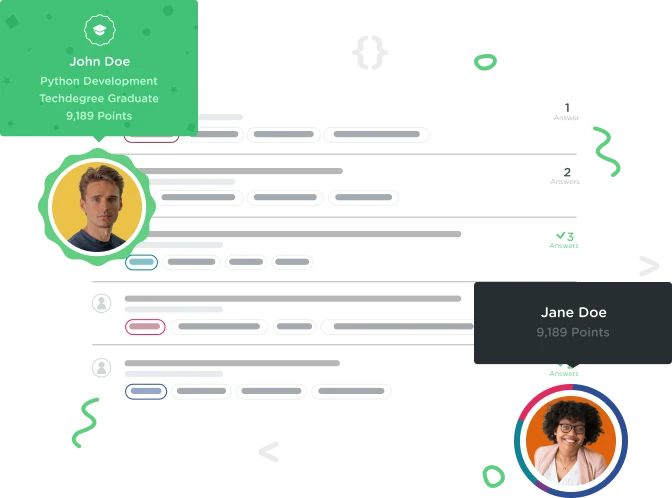

O'Ryan Alexander
Full Stack JavaScript Techdegree Student 5,671 PointsNot understanding what I have done wrong
When I click on check work it gives me this message "Bummer! You need to override the implementation for the fullName() method to pass the challenge", but I'm pretty sure I've done so.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
override func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Dr.", lastName: "Smith")
someDoctor.fullName()
3 Answers
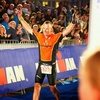
Steve Hunter
57,712 PointsHi there,
An overidden function needs to do something different to the function in the superclass. Yours doesn't at the moment.
You need to change the returned value from "Sam Smith" to "Dr. Smith" - that makes it different.
Try something like:
class Doctor : Person{
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Steve", lastName: "Hunter")
You don't need the init
method, either, as it is doing nothing different to the superclass version.
Steve.
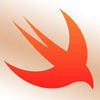
Jeff McDivitt
23,970 PointsYou need to override the function with the correct return that the challenge is asking for:
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Jeff", lastName: "McDivitt")
someDoctor.fullName()

O'Ryan Alexander
Full Stack JavaScript Techdegree Student 5,671 PointsThanks. I understand now what going on.
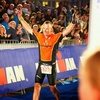
Steve Hunter
57,712 PointsGlad you got it. Shout if I can help with anything else.
Steve.