Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial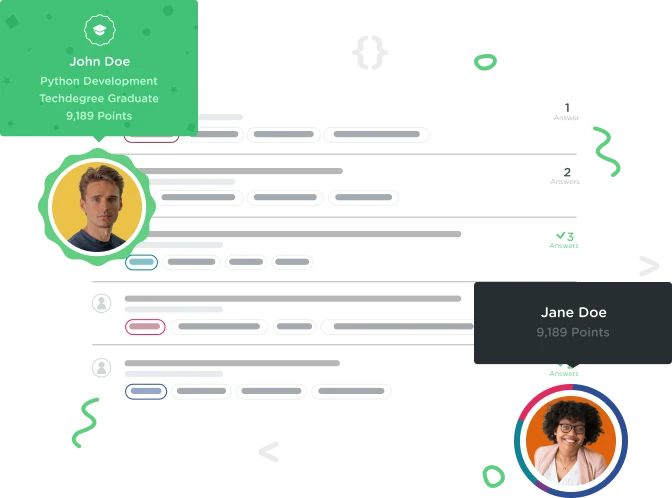

Cameron Andersen
2,649 PointsNot working
So don't we need a list to print a list? What is (items)? Is it a variable? Does it need a list to run? Seems like my code is okay but its not doing anything.
Also when you are printing a list such as
for x in items: print(x)
What is x? in relation to items, can it be anything? Whenever I look for an example of this x doesn't seem to be a variable or a declared variable, is there something I'm missing here? Thanks
def loopy(items):
items = ["apple", "orange", "STOP"]
for x in items:
print(x)
if items == "STOP":
break
3 Answers
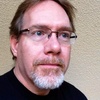
Chris Freeman
Treehouse Moderator 68,423 PointsYour code is headed in the right direction.
> So don't we need a list to print a list? What is (items)? Is it a variable? Does it need a list to run? Seems like my code is okay but its not doing anything.
items
is a formal parameter of the function loopy
. It is used as a reference to the argument used when calling the loopy()
function. If the function is called using loopy(['apple', 5, 'banana', 'STOP', 6])
then within the function code, items
would refer to the list ['apple', 5, 'banana', 'STOP', 6]
.
You do not have to define items
. The challenge checker will call your function with various values to test the code functionality.
> Also when you are printing a list such as for x in items: print(x)
, What is x? in relation to items, can it be anything? Whenever I look for an example of this x doesn't seem to be a variable or a declared variable, is there something I'm missing here?
In this case, x
is a local variable used to hold the next value for each item in the list items
. For the data above, x
would be "apple
" in the first iteration, then 5
, then, "banana
", etc.. The name of this loop variable is up to the coder to pick something meaningful. In this case, "item" might be better, as in for item in items:
which can be read as "for each item in the collection items, do...
".
Here is your code corrected:
def loopy(items):
# items provided in argument list. Do not define within function
# items = ["apple", "orange", "STOP"]
for x in items:
# indent 'if' to be inside of for-loop
# check if loop variable 'x' is "STOP"
if x == "STOP":
break
# move print after 'if' so printing stops before STOP is printed
print(x)
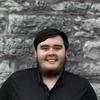
Michael Hulet
47,912 PointsYes, you do need a list in order to print one, but you don't need to make one yourself, because it will be handed to you with the name "items
" when the loopy
function runs. You can treat items
as you would any other variable, but you don't need to define it first, because it will already be defined when the loopy
function runs. For example, consider this line of code:
loopy(["This", "is", "a", "list"])
In this case, items
will be a list equal to ["This", "is", "a", "list"]
. You can pass the loopy
function anything you want as I did above, and whatever you write in there between the parentheses will be accessible inside of the loopy
function as "items
"
A for
loop in Python does need some kind of iterable to run, such as a list. A for
loop performs an action on each member of an iterable (in this case, a list), and x
will always be equal to the current item inside of the iterable that the loop is operating on. For example, consider this code:
for x in ["This", "is", "an", "iterable"]:
print(x)
The first time the loop runs, x
will be "This"
, so "This"
will be printed to the console. On the next iteration, x
will be "is"
, so "is"
will be printed to the console. The next time x
will be "an"
, and the last time x
will be "iterable"
.
The reasons your code probably isn't passing the challenge is because you're manually defining the items
variable, and because the condition to break
the loop if the value is "STOP"
is out of scope of the loop itself. This worked for me:
def loopy(items):
# Notice how I never define the items variable
for x in items:
# The condition to break the loop should come before you print the item
if x == "STOP":
break
else:
print(x)

Cameron Andersen
2,649 PointsThank you for you responses, this helps a lot.