Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial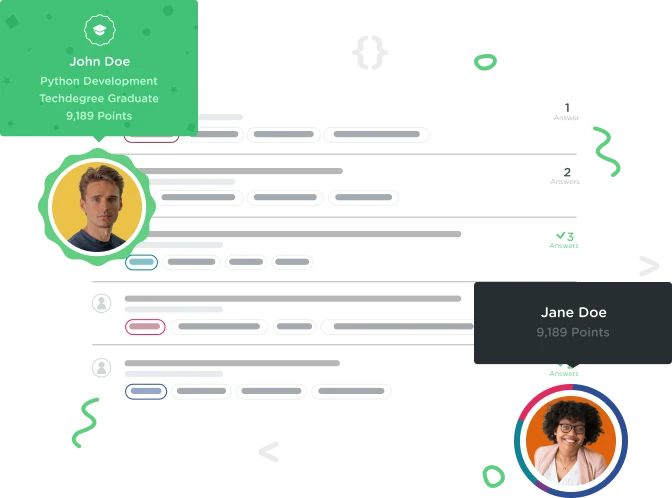

akshaan Mazumdar
997 PointsNot Working. Why?
what is the issue with the code
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item(box):
x = random.randint(0,len(box)-1)
return box.index(x)
2 Answers

andren
28,558 PointsThe problem is your use of the index
method. index
is used to find the index of a particular value in a list. It is not used to retrieve a value. To retrieve the value you can simply use bracket notation like this:
import random
def random_item(box):
x = random.randint(0,len(box)-1)
return box[x]

Mike Wagner
23,559 PointsThe index()
method in Python is designed to return the first index at which a member is found. This means that, in the way you're using it, you are assigning a random number to x
which is then being looked for in the string. This will result in a TypeError, since you cannot implicitly convert an int
to a str
. Though, ignoring that, if you were to cast the x
to string by using str(x)
instead, it's worth mentioning that you'll usually receive a ValueError, as you will not find the substring of a random number x
within a string "Treehouse
" or similar strings. In your case, what the Challenge is actually asking for is the member value, which means you should reference your iterable box
and pass in the known index x
as it's parameter (i.e. return box[x]
).