Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial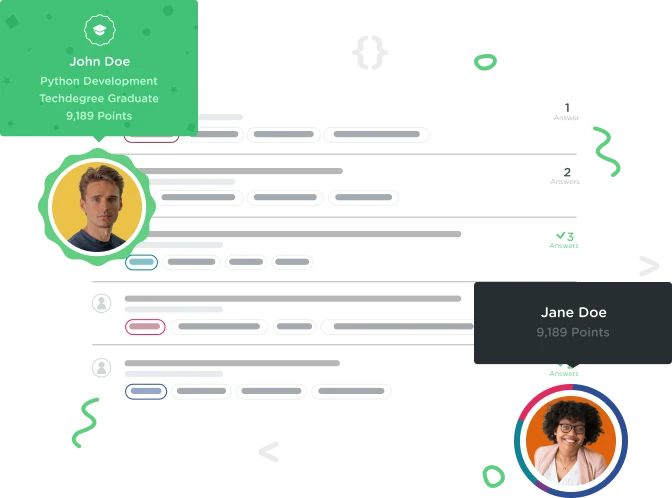

Minuet Kurjakovic
2,459 PointsNothing happens when I click submit
Hello, I can't figure out what's wrong with my code. Can someone please help?
index.html:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, minimum-scale=0.5, maximum-scale=0.5, minimal-ui">
<title>MASH</title>
<link href="normalize.css" rel="stylesheet">
<link href="style.css" rel="stylesheet">
</head>
<body>
<h1 class="logo"><img src="mash1.png"></h1>
<p class="description">Fill in the blanks and your future will be foretold!</p>
<form action="" method="post" id="mash">
<div id="answers" class="hide">
<p>Your home will be painted <span id="color"></span> with a <span id="pet"></span> and a <span id="car"></span> in the front of the <span id="home"></span>.
</div>
<div class="bucket">
<div class="choice-bucket">
<h4 class="highlight">What is your favorite primary color?</h4>
<input name="color[]">
<input name="color[]">
<input name="color[]">
<input name="color[]">
</div>
<div class="choice-bucket">
<h4 class="highlight">How many pets do you want to have?</h4>
<input name="pet[]">
<input name="pet[]">
<input name="pet[]">
<input name="pet[]">
</div>
<div class="choice-bucket">
<h4 class="highlight">What is your favorite type of car?</h4>
<input name="car[]">
<input name="car[]">
<input name="car[]">
<input name="car[]">
</div>
</div>
<input type="submit" value="Tell my fortune">
</form>
<script src="script.js"></script>
</body>
</html>
script.js:
function random_number(num) { // New function called random_choice that takes one parameter, num (or a number)
// Get a random number between 0 and a passed-in number
var num = num || 4 // If no number passed in, default to 4
return Math.floor(Math.random() * num); // Round the answer down (floor) of a random number between 0 and 1 and multiply it by a number. Then return a value and exit the function.
}
function mash_choice() { // New function called mash_choice that doesn't take any parameters
// Since MASH is a special case, give it its own list
var mash = ['mansion', 'apartment', 'shack', 'house']; // The array of choices to pick from
var randomNum = random_number(4); // Use the above function to get a number between 0 and 4
return mash[randomNum]; // Return the list item the random number function just picked and exit the function
}
function get_answer(category) {
// Get a random answer from the available answers in a given category
var choices = []; // A blank array to hold the user provided answer
var selector = 'input[name="' + category + '[]"]'; // Build a CSS selector for the blanks in our passed in category
var inputs = document.querySelectorAll(selector); // Get all of the inputs that match our selector
var answer;
for (var i = 0; i < inputs.length; i++) { // Begin a for loop that will run through the code. i++ = add one to the counter which is "i"
answer = inputs[i].value; // Get the input with the index value of the counter and get the value ie. if they typed in dog, you get back "dog"
if (answer !== '') { // If answer doesn't equal a blank... !== means doesn't equal
choices.push(answer); //...add it to the end of the list
}
}
return choices[random_number(choices.length)]; // Pick and return a random choice choice.length = number of answers the user provided in that category
}
function fill_in_answers(answers) {
// Find the spans that need filled
var home = document.querySelector('#home'); // This says make a new variable and find the HTML tag that has the ID of "home"
var color = document.querySelector('#color');
var pet = document.querySelector('#pet');
var car = document.querySelector('#car');
// Fill them with the provided answers
home.innerText = answers['mash'];
color.innerText = answers['color'];
pet.innerText = answers['pet'];
car.innerText = answers['car'];
home.innerHTML = answers.mash; // Change the content of the element in the HTML doc with the id "home" to the "mash" value in answers
color.innerHTML = answers.color; // Change the content of the element in the HTML doc with the id "career" to the "career" value in answers
pet.innerHTML = answers.pet;
car.innerHTML = answers.car;
}
function handle_submission(evt) {
evt.preventDefault(); // Stop the form from reloading the page
evt.stopPropagation(); // Stop the form from reloading the page
// Build up our answers object
var answers = {
'mash': mash_choice(),
'color': get_answer('color'),
'pet': get_answer('pet'),
'car': get_answer('car')
}
// Fill in the answers
fill_in_answers(answers);
var answer_div = document.querySelector('#answers');
answer_div.classList.add('show');
}
// Find the form on the page and attach a handler for when it's submitted
var form = document.querySelector('#mash');
form.addEventListener('submit', handle_submission); // Anytime the form is submitted, we want to call the function handle_submission
Have questions about this video? Start a discussion with the community and Treehouse staff.

Minuet Kurjakovic
2,459 Pointslol well that's frustrating. I don't know what I'm doing wrong then... All of my files are named correctly and they are all stored in the same folder.
2 Answers
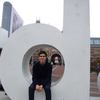
Nick Falcone
10,832 PointsMy output was similar to Helmut Granda's. I would try this:
- Comment-out the last line of the script.js file using // (this and with are both reserved words in JavaScript)
- If you are editing the files outside of workspaces, use the full link for the JavaScript file. You can right-click in most text editors and select "copy path." It could look like this: Users/yourname/downloads/script.js Use this link in the script tag: <script src="Users/yourname/downloads/script.js"></script>
- Make sure in your browser settings that JavaScript is enabled.

Minuet Kurjakovic
2,459 PointsThank you for the input. It made me think that the issue was the folder path that I have set up, so I created a subfolder, named it java, and then changed the code to <script src="java/script.js"></script> and now it works!!
Thanks again!

Gonras Karols
17,357 PointsTry to <script src="./script.js"></script>

Minuet Kurjakovic
2,459 PointsThank you for your help. I copied/pasted your suggestion and nothing is coming up, still. When you try the webpage, does it work for you?
Helmut Granda
10,343 PointsHelmut Granda
10,343 PointsIs it maybe the way you are sourcing the external files? I tried the code you posted on this page and it works. I got the following in my fist try:
"Your home will be painted Blue with a 1 and a three in the front of the house."