Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial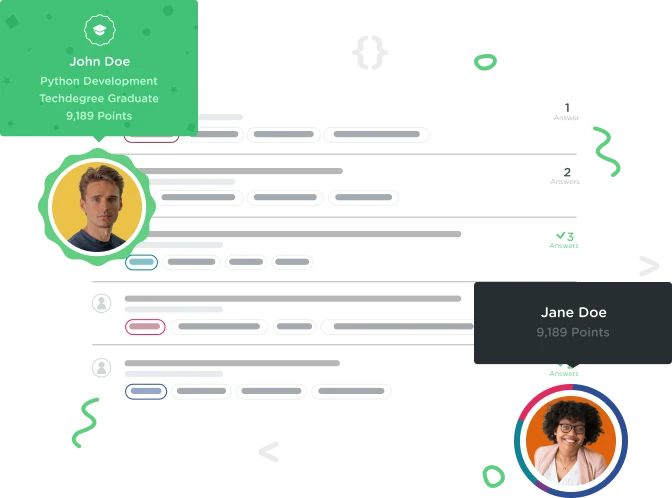
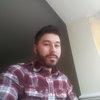
Henry Brenton
10,144 PointsNothing is printing to the console - I've checked my code several times, can't see anything wrong with it
Hi all,
I've followed the code in this video, and checked it several times, so am confident my code is correct, however, nothing prints to the console when I run the program, in either case for the indices.
Please could I have some help in understanding what the problem is?
My code is as follows:
def linear_search(list, target): """ Returns the index position of the target if found, else returns None """
for i in range(0, len(list)):
if list[i] == target:
return i
return None
def verify(index):
if index is not None:
print("Target found at index: ", index)
else:
print("Target not found in list")
numbers = [1,2,3,4,5,6,7,8,9,10]
result = linear_search(numbers, 12)
verify(result)
result = linear_search(numbers, 6)
verify(result)
Many Thanks
Henry
2 Answers

KRIS NIKOLAISEN
54,972 PointsFrom the above it looks like your for loop lines up with def verify. It needs to be indented
def linear_search(list, target):
"""
Returns the index position of the target if found, else returns None
"""
for i in range(0, len(list)):
if list[i] == target:
return i
return None
def verify(index):
if index is not None:
print("Target found at index: ", index)
else:
print("Target not found in list")
numbers = [1,2,3,4,5,6,7,8,9,10]
result = linear_search(numbers, 12)
verify(result)
result = linear_search(numbers, 6)
verify(result)

Marcel Durgante Ramos
79 PointsHey team,
I am using Javascript. How can I output to the console the result of my code?
I have tried: node linear_search.js and node linear_search but nothing returns.
Thank you for your help.
Henry Brenton
10,144 PointsHenry Brenton
10,144 PointsIndeed - silly me, thank you! I need to readjust to this whole 'indentation' thing