Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial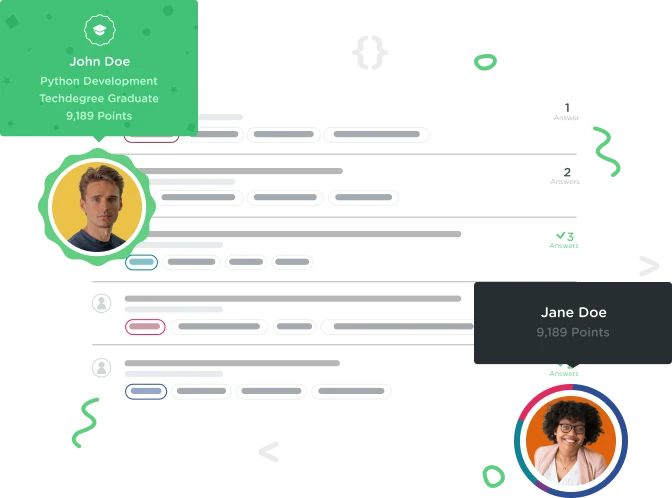

isaac schwartzman
963 PointsNow add a constructor that accepts author, title, body, category and creation date. In your constructor, initialize the
its saying my Treet constructor is wrong and idk what I did.
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
}
public Treet(String title, String author,String body,String category,Date creationDate){
mTitle = title;
mAuthor = author;
mBody= body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor(){
return mAuthor;
}
public String getTitle(){
return mTitle;
}
public String getBody(){
return mBody;
}
public String getCategory(){
return mCategory;
}
public Date getCreationDate(){
return mCreationDate;
}
1 Answer

andren
28,558 PointsThere are a couple of issues:
You have placed the constructor and the getters outside the body (the {} brackets) of the class. Which is not correct since they belong to the class.
A constructor has to be named exactly the same as the class it is placed in, since the class is named BlogPost the constructor has to be named that too. So
Treet
is not an acceptable name.
Also while not a direct issue you have added way more code than was asked for. Task 2 only asks you to add a constructor, not to add getters for all the properties. Task 3 does ask you to add a getter but only for the body
field. Adding more code than is asked for can often lead to issues as the code checker for these challenges tend to be pretty strict. So I'd advise not doing so in the future.
If you fix the issues I have outlined like this:
package com.example;
import java.util.Date;
public class BlogPost { // This starts the body of the BlogPost class
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String title, String author,String body,String category,Date creationDate){
mTitle = title;
mAuthor = author;
mBody= body;
mCategory = category;
mCreationDate = creationDate;
}
public String getBody(){
return mBody;
}
} // This ends the body of the BlogPost class
Then your code will pass the challenge.