Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial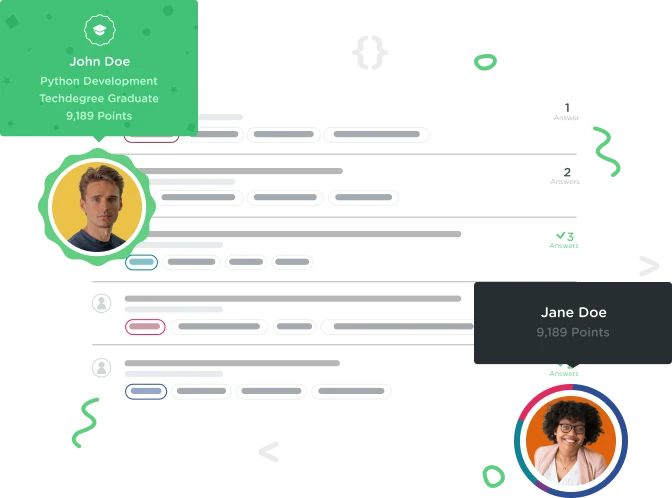

MUZ140796 Sadson Mkumira
4,308 PointsNow add a second constructor that takes a String parameter. Use this parameter to update mType to a specific type of spa
I'm stuck here. Please help!
public class Spaceship {
public String mType = "ROCKET";
public Spaceship (String type, Rocket) {
mType = "ROCKET";
}
public Spaceship () {
mType = "SHUTTLE";
}
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
1 Answer
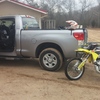
Ryan Ruscett
23,309 PointsYou you are a bit off here. Let me try to explain inside code example.
TASK 1 - create a constructor that takes 0 arguments and sets mType to SHUTTLE.
//You set mType to SHUTTLE, in the first no arg constructor. This is so that if I call an instance of this class to create an object, //and I don't give it any params. it will auto default and create an object of a spaceship called SHUTTLE.
//This is the class and I create a variable mType. That's it. No value or anything. Like so below:
public class Spaceship {
public String mType;
}
// first no arg constructor. I set mType = to SHUTTLE. So when I do 'Spaceship X = new Spaceship();' I get a spaceship with the //name of SHUTTLE. Like so below.
public Spaceship () {
mType = "SHUTTLE";
}
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
EXERCISE TWO
Now add a second constructor that takes a String parameter. Use this parameter to update mType to a specific type of spa
// Create a variable for mType, Check!
public class Spaceship {
public String mType;
//Create first no arg constructor so if I pass in no values when creating the object it gets called SHUTTLE by default
public Spaceship() {
mType = "SHUTTLE";
}
//Add a second constructor. So if I don't want a default value of SHUTTLE. I can do 'Spaceship X = new Spaceship("ROCKET");'
// Now because of the method signature. It will not call the no arg constructor, it will call the constructor that matches //arguments passed in. Then set mType to whatever is passed in. I created the variable but did not define it at the
//class level so it would be available to set in different methods so scope isn't an issue. It's not static or final so I don't have
// to set it right off the bat. This comes into play more so when member variables are private but out of the scope of the
//question lol
public Spaceship(String type) {
mType = type;
}
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
I hope that helps, let me know if you have any other questions I can try to clear up.
Thanks!
MUZ140796 Sadson Mkumira
4,308 PointsMUZ140796 Sadson Mkumira
4,308 PointsThank u so much, it worked when i used the String text...