Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial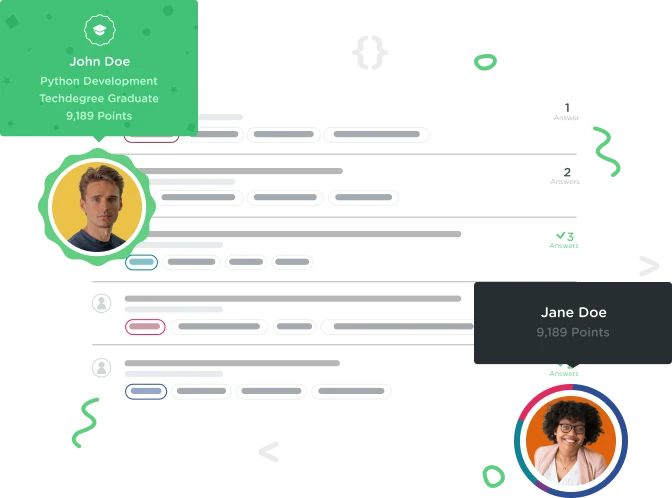
Peter Price
4,007 PointsNow create a helper method called isFullyCharged. It should return true if the GoKart is at max charge.
Hi, I have a problem with the task written above. I would be grateful if someone could help.
This is the compiler's error code:
GoKart.java:16: error: illegal start of type if (!goKart.isFullyCharged()){ ^ ./GoKart.java:16: error: illegal start of type if (!goKart.isFullyCharged()){ ^ ./GoKart.java:16: error: ')' expected if (!goKart.isFullyCharged()){ ^ ./GoKart.java:16: error: ';' expected if (!goKart.isFullyCharged()){ ^ ./GoKart.java:16: error: invalid method declaration; return type required if (!goKart.isFullyCharged()){ ^ ./GoKart.java:16: error: ';' expected if (!goKart.isFullyCharged()){ ^ 6 errors
My code is below:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
GoKart goKart = new GoKart ("red");
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean isBatteryEmpty(){
return mBarsCount == 0;
}
public boolean isFullyCharged(){
return mBarsCount == 0;
}
if (!goKart.isFullyCharged()){
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
}
1 Answer
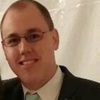
Jeremiah Montano
7,221 PointsI believe your code may be structured incorrectly. You have declared a new class outside of a method on line 5. Your method for isFullyCharged stops on the first line which says return. The IF line appears to also be outside a method so that is why you are receiving the error. If i remember this exercise correctly, the code for this should look something like this:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public boolean isBatteryEmpty(){
return mBarsCount == 0;
}
public boolean isFullyCharged(){
return mBarsCount == MAX_BARS;
}
public String getColor() {
return mColor;
}
public void charge() {
if (!goKart.isFullyCharged()){
mBarsCount = MAX_BARS;
}
}
}
I don't remember the exact details but remember that anything you declare should be inside a method. Initializing a new class, such as the GoKart gokart = new GoKart("Red"); should reside only in the main method and not the class method. I hope this helps!
Peter Price
4,007 PointsPeter Price
4,007 PointsHi Jeremiah, Thanks for your reply. I tried your code but it says: "There is a compiler error. Please click on preview to view your syntax errors." When I click on preview, it shows this:
GoKart.java:21: error: cannot find symbol
if (!goKart.isFullyCharged()){
(arrow)
symbol: variable goKart
location: class GoKart
1 error
Does anyone (including Jeremiah) have any idea of what it wants changed?