Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial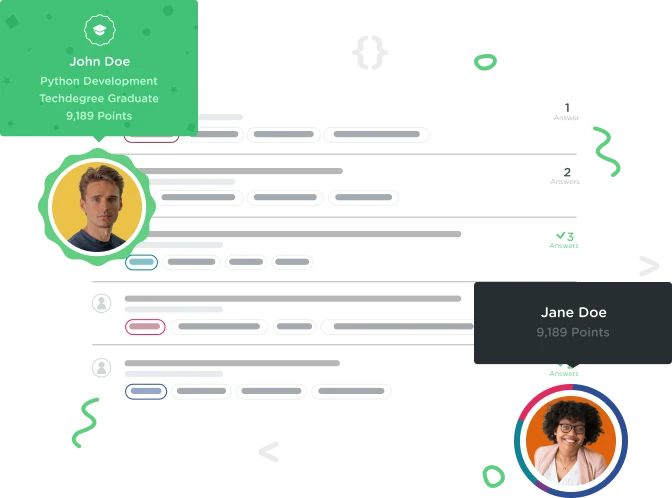

Zack Parashar
1,007 PointsNow let's create a similar method named isFullyCharged that checks to see if the current bar count is at the maximum cha
Not sure what I did wrong.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
if (barCount == 0) {
return true;
} else {
return false;
}
}
public boolean isFullyCharged() {
return BarsCount == MAX_BARS;
}
}
5 Answers
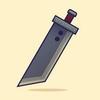
Allan Clark
10,810 Pointscheck you capitalization and spelling on the variable names on this line
return BarsCount == MAX_BARS;

jasonewillis
1,431 PointsI guess I thought conditional statements, by default, are true. So, if we replace (barCount == MAX_BARS) with (true) || (false), depending on how they evaluate, to me it should still work. If true, return true. If false, skip code block. In the original, I believe I had 'return false' after the conditional code block.
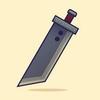
Allan Clark
10,810 PointsIf I'm understanding correct, yes this should be logically equivalent to the accepted answer
public boolean isFullyCharged() {
if (barCount == MAX_BARS) {
return true;
}
return false;
}
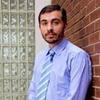
Zach Freitag
20,341 PointsSpoiler Alert!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
if (barCount == 0) {
return true;
} else {
return false;
}
}
public boolean isFullyCharged() {
if (barCount == MAX_BARS) {
return true;
}
return false;
}
}

jasonewillis
1,431 PointsI did have a similar but seemingly incorrect code try:
public boolean isFullyCharged() {
if (barCount == MAX_BARS) {
return true;
}
Wondering why this logic produced an error.
I did like the way Allan and Zack wrote the logic though. Less redundant than my attempt. ;)
// return (result of whether a comparison of 'barCount == MAX_BARS' is true or false)
Thanks you!
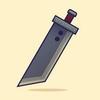
Allan Clark
10,810 PointsIt's because you need to tell the method what to return on every branch of your logic tree. If the condition is not met the method does not have a return statement, so it doesnt know what to do when barCount == MAX_BARS evaluates to false. Add 'return false;' outside the if statement and this should compile fine.

Ryan Ferguson
649 PointsI was able to get this to work with the following:
public boolean isBatteryEmpty () { return barCount == 0; }
public boolean isFullyCharged () { return barCount == MAX_BARS; }
I did not have to tell it to return true/false or write an if statement.
Allan Clark
10,810 PointsAllan Clark
10,810 Pointsif this doesn't solve it please post the error you are getting.
Zack Parashar
1,007 PointsZack Parashar
1,007 PointsThanks!