Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial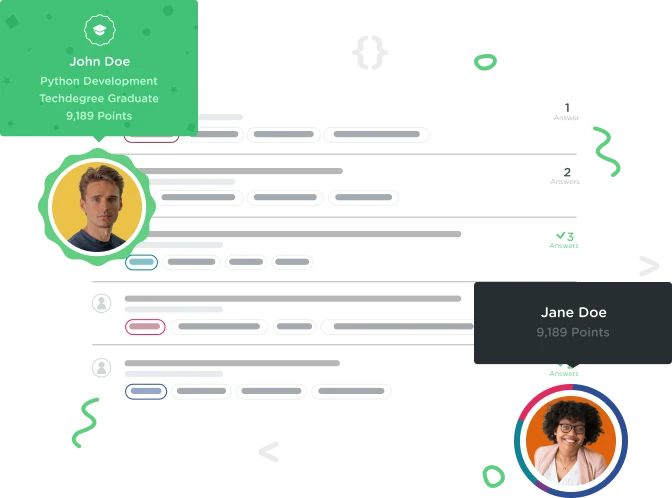

Tal Reznic
4,633 PointsNow, make a function named summarize that also takes a list. It should return the string "The sum of X is Y.", replacing
How is it possible to get back form list of numbers a string
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
def add_list(list):
total = 0
for item in list:
total = total + item
return total
def summarize(list):
total = 0
string_version = str(" ".join(list))
for item in list:
total = total + item
return "The sum of {} is {}".format(string_version,total)
3 Answers
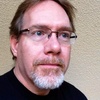
Chris Freeman
Treehouse Moderator 68,423 PointsFiguring out the sum of the list again in summary
is missing the point. You have a function to do this: add_list()
.
def add_list(lst):
total = 0
for item in lst:
total = total + item
return total
def summarize(lst):
return "The sum of {} is {}".format(lst, add_list(lst))
Also, note that I changed the variable to lst
so as to avoid using the reserved word list
. I also increased the indention to 4 spaces.
Also note that your original code passes if you change the string_version
line to:
string_version = str(list)
Or by simply letting the format
method convert it for you.

Bryan Laraway
13,366 PointsActual, for this task you would just return the list as the first variable in the formatted string, like this:
def add_list(list):
total = 0
for item in list:
total = total + item
return total
def summarize(list):
total = 0
for item in list:
total = total + item
return "The sum of {} is {}".format(list,total)
No need to convert the list itself to a string!

Charles Ratkie
11,607 Pointsdef add_list(a_list):
for item in a_list:
return sum(a_list)
def summarize(b_list):
return ("The sum of {} is {}".format(b_list, sum(b_list)))
Basically Chris is using 'his own' sum function, which is helpful to understand how it works. Same idea, but I just used the built in sum function within python to achieve the same result. Also, the name isn't as important for the argument just as long as you use the same name within your function.
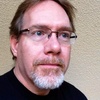
Chris Freeman
Treehouse Moderator 68,423 PointsThis challenge is from early on in the Python Basics course. One of the intended purposes of this challenge is to teach how for loops work by building your own function. The built-in sum()
has not yet been introduced. The other intent is to introduce the concept of calling one function from within another function.

Charles Ratkie
11,607 PointsThanks Chris, you are right!