Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial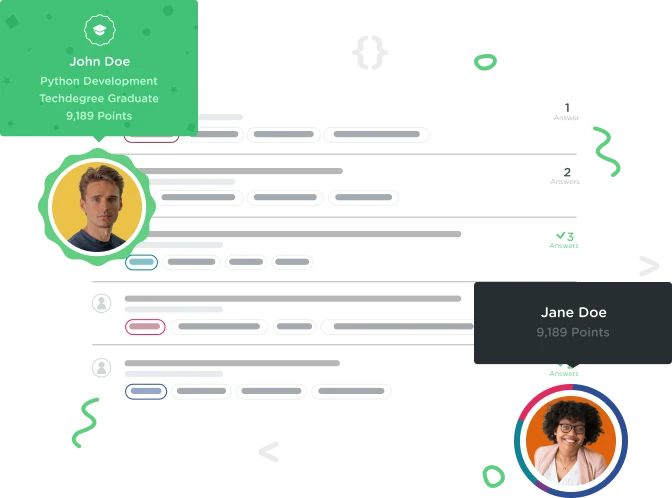
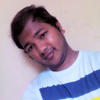
ambareesh M
6,122 PointsNow make sure that if a com.example.BlogPost is passed in for obj that you then cast it to a BlogPost. Return the result
no idea
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result ="";
if(obj instanceof String)
result =(String)obj;
return result;
}
}
3 Answers
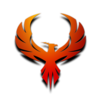
Brian Pedigo
26,783 PointsStuart is right. The code to pass this challenge should look like the code below. I am sure this isn't the only way to pass it but it works. The main trick to the second part is to type cast the obj parameter to BlogPost object so that you can use it's getTitle method which we know returns a string. I hope this helps! Happy coding!
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if(obj instanceof String){
result = (String) obj;
}
if(obj instanceof BlogPost){
BlogPost post = (BlogPost) obj;
result = post.getTitle();
}
return result;
}
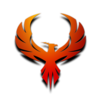
Brian Pedigo
26,783 PointsQ: elaborate as to why you need to make an BlogPost variable first ?
A: This is a characteristic of a strongly typed programming language like java, C++, C , C# etc.. Where you have to declare your variables or the compiler/virtual machine will throw errors. Java is a strongly typed programming language because every variable must be declared with a data type. A variable cannot start off life without knowing the range of values it can hold, and once it is declared, the data type of the variable cannot change.
Q: It gave me the error of cannot find symbol the getTitle method. Anything i'm overlooking as to why obj cannot access the method ?
A: The main point of the lesson is that the obj variable can be anything, and that you need to cast it to fit your goals. When the "if (obj instanceof BlogPost) returns true " the JVM doesn't automatically cast obj to a BlogPost object because Java is a strongly typed language. Therefore, you have to cast it to a BlogPost object in order to use the getTitle method.
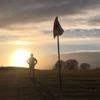
Stuart Wright
41,118 PointsThe second part of the challenge isn't all that different from the first part of the challenge, which you've managed to complete. You still want to follow similar steps:
- a conditional block to check if your variable is an instance of a certain type.
- if so, cast your object to that type and set the result variable to the appropriate value.
The main difference is that the second part above should be split across two lines:
- first line should be to create a new BlogPost object by casting obj to type BlogPost.
- second line should be to call the getTItle() method on your new BlogPost object, and assign the value of that to result.
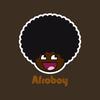
adsdev
15,583 PointsCan Brian Pedigo or Stuart Wright elaborate as to why you need to make an BlogPost variable first ?
What I did, and which did not work , is this :
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if(obj instanceof String){
result = (String) obj;
}
if(obj instanceof BlogPost){
obj = (BlogPost) obj;
result = obj.getTitle();
}
return result;
}
Add Comment
It gave me the error of cannot find symbol ath the getTitle method. Anything i'm overlooking as to why obj cannot acces the method ?