Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial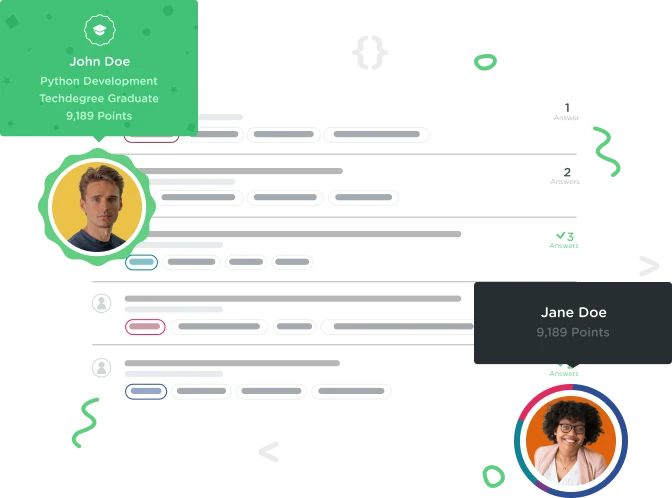
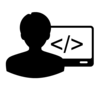
Abe Layee
8,378 PointsNow set up the prototype chain for the Teacher prototype to inherit from the Person prototype
This is pretty much one of the difficult course I have ever taken on JavaScript. From the start to the end is all confusing to me. I have no clue what I am even doing or what the challenge is asking. I can't do the challenge if I don't understand what is goind on. I need to do some research on this JavaScript Object Oriented programming or read a book on it.
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.fullName = function() {
return this.firstName + " " + this.lastName;
};
function Teacher(firstName, lastName, roomNumber) {
Person.call(this,firstName,lastName)
this.room = roomNumber;
}
Teacher.prototype = Object.create(person.prototype)
3 Answers
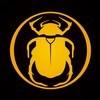
rydavim
18,813 PointsDisclaimer: I'm not an expert on this topic (I'm taking this course right now), but I'll do my best.
For the first part of the challenge, you're using call() to chain constructors that share common attributes. Because a Teacher is very similar to a Person, you can use Person to help shorten the Teacher constructor.
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
// this.firstName = firstName; // You don't need these lines anymore.
// this.lastName = lastName; // The Person call is doing this for you.
this.room = roomNumber;
}
In this example, it's not that big a change. But you can call the Person constructor on lots of kinds of people, assuming you had constructors for them. What if you had Teachers, Students, Staff, Mods, ect. It would be convenient to reuse the Person call for all of them, yeah?
Edit - Added the second part of the challenge.
For the second part of the challenge, you're setting Teacher's prototype to Person's constructor, meaning you inherit all of Person.prototype's properties and methods.
Teacher.prototype = Object.create(Person.prototype);
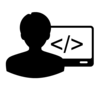
Abe Layee
8,378 Pointsso what about the second part of the challenge. I have no idea what to do.
function Teacher(firstName, lastName, roomNumber) {
Person.call(this,firstName,lastName)
this.room = roomNumber;
}
Teacher.prototype = Object.create(person.prototype)
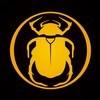
rydavim
18,813 PointsI've added some additional information to my original post.
If you're interested, there is a pretty good article on JavaScript prototypes at javascriptissexy.com that I found pretty helpful.
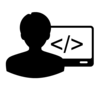
Abe Layee
8,378 PointsThank you rydavim. I was just reading JavaScript OOP on that website you showed .