Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial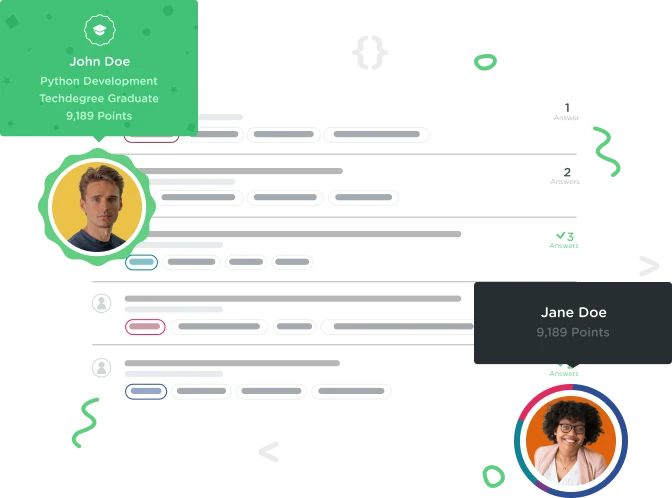

Zubeyr Aciksari
21,074 PointsNow switch to the ArtistActivity.java file. This also has tabs, but we are going to use icons instead of text. Set the i
Hi, i am confused in here, please help! Thanks! "Now switch to the ArtistActivity.java file. This also has tabs, but we are going to use icons instead of text. Set the icon for each tab using the setIcon() method, and use the value returned by the PagerAdapter's getIcon() method as the parameter for setIcon()."
import android.app.ActionBar;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
public class MainActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
/*
* Add your code here to set the text!
*/
tab.setText(pagerAdapter.getPageTitle(i));
mActionBar.addTab(tab);
}
}
// These listener methods are intentionally blank
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
}
import android.app.ActionBar;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
public class ArtistActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_artist);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
/*
* Add your code here to set the icon!
Set the icon for each tab using the setIcon() method,
and use the value returned by the PagerAdapter getIcon() method as the parameter for setIcon().
*/
getIcon()
mActionBar.addTab(tab);
}
}
// These listener methods are intentionally blank
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
}
import android.content.Context;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
public class PagerAdapter extends FragmentPagerAdapter {
/*
* Some code has been omitted for brevity!
*/
public PagerAdapter(FragmentManager fm) {
// code omitted
}
@Override
public int getCount() {
return 3;
}
@Override
public CharSequence getPageTitle(int position) {
switch (position) {
case 0:
return "Songs";
case 1:
return "Albums";
case 2:
return "Artists";
}
return null;
}
public int getIcon(int position) {
switch (position) {
case 0:
return R.drawable.ic_tab_profile;
case 1:
return R.drawable.ic_tab_discography;
case 2:
return R.drawable.ic_tab_related_artists;
}
return R.drawable.ic_tab_profile;
}
}
2 Answers
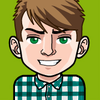
Harry James
14,780 PointsHey Zubeyr!
On this question, it greatly helps if we take a look at PagerAdapter
's getIcon()
method:
public int getIcon(int position) {
// code
}
Here, we can see that it takes in a position as an integer.
So, back in ArtistActivity.java
, we want to set the icon for each tab. So, we're going to need to use the setIcon()
method and we want to set the value to what is returned by getIcon().
So, how do we return the right image? Well, take a look at the for loop. Is there something that we can use to get a position? Hint:
for (int i = 0; i < pagerAdapter.getCount(); i++) {
// code
}
I'll leave the final putting together for you to do. Hopefully this should get you started and help you understand the question but, if there's still something you're stuck on then please give me a shout :)

Zubeyr Aciksari
21,074 PointsI didn't understand this Harry.. i tried this but didn't work:
getIcon(setIcon(onTabSelected));
getIcon(setIcon(onTabUnselected));
getIcon(setIcon(onTabReselected));
Please further guidance! Thanks!
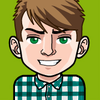
Harry James
14,780 PointsIt's almost exactly like the first part of the Code Challenge - you're running setIcon() off the tab variable and for the parameter of setIcon(), you want to put the getIcon() method.
Note that onTabSelected()
, onTabUnselected
and onTabReselected
aren't integers and so, this method would fail with them. In the parentheses of getIcon()
, you should put the position which is an integer (Hint! Take a look at the for loop like I said in my last answer! There's a position available for you to use here if you know how to get it!).
So, you should be following this in the following order:
- tab
- getIcon()
- setIcon()
Hopefully this should guide you along but, if you're still stuck, I'll start you off.