Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial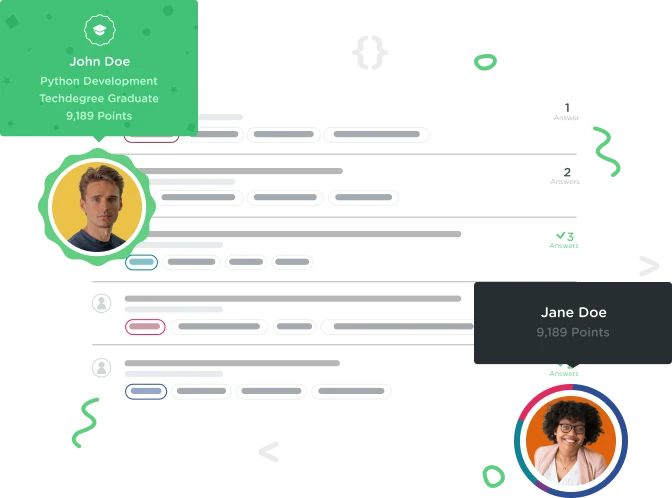
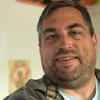
Jeff Ripke
41,989 PointsNow that we have an instance of UIColor as a stored property, let's change the background color of the backing view.
This does not make sense to me. What is the backing view?
class ViewController: UIViewController {
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
let blueColor = UIColor(red: 0/255.0, green: 187/255.0, blue: 181, alpha: 1.0)
.backgoundColor = blueColor
}
5 Answers
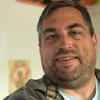
Jeff Ripke
41,989 PointsNot sure what backing view is, does not make sense to me. I try backing.backgroundColor = blueColor and that does not work.
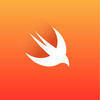
Steven Deutsch
21,046 PointsHey Jeff Ripke,
First, if you're going to create an instance of UIColor, you need to use the values specified in the challenge. These are (0, 0, 255, 1.0) respectively. Second, I recommend placing this instance above viewDidLoad and setting the backing view inside of viewDidLoad. You can set the backgroundColor of the backing view by using view.backgroundColor.
class ViewController: UIViewController {
// The challenge is picky, it only accepted an Int of 255 for blue
let blueColor = UIColor(red: 0/255.0, green: 0/255.0, blue: 255, alpha: 1.0)
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
view.backgroundColor = blueColor
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
OR you could solve it using a convenience method for a blue UIColor
class ViewController: UIViewController {
// Blue color convenience method approach
let blueColor = UIColor.blueColor()
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
view.backgroundColor = blueColor
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
Good Luck!

Adam Petritsis
2,942 PointsWhy do we need to put "view.backgroundColor = blueColor" inside viewDidLoad and not in the end?
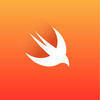
Steven Deutsch
21,046 PointsHey Adam Petritsis,
We need to put the code inside of viewDidLoad because this is where we do any additional setup for the UIViewController. Behind the scenes, UIKit will call this method and apply whatever changes are inside of it to the ViewController. You can also place this code inside of viewWillAppear()
.
Good Luck
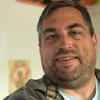
Jeff Ripke
41,989 PointsNot sure how I messed that up, but that was not really my question. The question was about the second part: "Now that we have an instance of UIColor as a stored property, let's change the background color of the backing view. Access the view controller's backing view and assign the UIColor instance to the view's backgroundColor property."
What is the view controller's backing view?
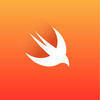
Steven Deutsch
21,046 PointsYour view controller is linked to a view in the storyboard. You're accessing the view linked to the view controller you are in and changing the color of the view's background to blue. That is what view is referring to here. You access the backgroundColor property of that view and set it to a different color.

Rich Braymiller
7,119 Pointswow just when i thought i was making progress...im in la la land again...
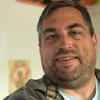
Jeff Ripke
41,989 PointsThanks Steven Hunter that makes sense, I did do google search and could not find anything.
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsThe backing view is the view in your Main.storyboard file that is linked to your view controller you are writing your code in. This is referenced as "view". That is the backing view. The view in your storyboard file that is backing your ViewController. So we use view to reference this, then access the backgroundColor property of view, and set it to the color that we want.
Makes sense?