Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial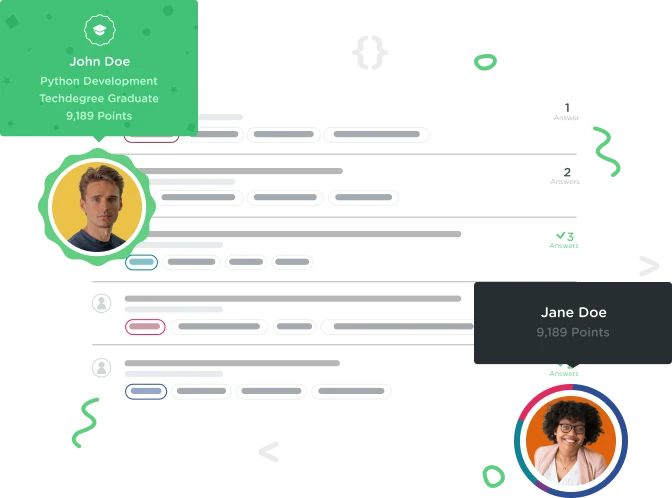

Matthew Wadley
1,282 PointsNow that we know about Generics, let's upgrade our Comparable interface defined on the BlogPost class to use it. Rememb
I have no idea how to do this, please help.
package com.example;
import java.io.Serializable;
import java.util.Date;
public class BlogPost implements Comparable, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(Object obj) {
BlogPost other = (BlogPost) obj;
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("[^\\w']+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
10 Answers

Dan Johnson
40,533 PointsTo specify type with generics, you use angle brackets:
Comparable<BlogPost>
Specifying BlogPost
for Comparable
will let you update the parameter for the compareTo method as well as get rid of the down cast as it will now be expecting a type of BlogPost
.

Maria Kochetygova
Courses Plus Student 1,784 Pointspublic int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}

k doshi
128 PointsWhy not public int compareTo<BlogPost other>
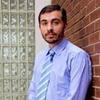
Zach Freitag
20,341 PointsSpoiler Alert!
package com.example;
import java.io.Serializable;
import java.util.Date;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("[^\\w']+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}

k doshi
128 PointsWhy not public int compareTo<BlogPost other>
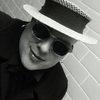
Erik Sorensen
13,846 PointsYou need to make changes in two locations -- be sure to pay attention to camel case.
public class BlogPost implements Comparable<BlogPost>, Serializable {
@Override public int compareTo(BlogPost other) { BlogPost other = (BlogPost) obj; if (equals(other)) { return 0;

k doshi
128 PointsWhy not public int compareTo<BlogPost other>

Maria Kochetygova
Courses Plus Student 1,784 PointsComparable<BlogPost>
@Override public int compareTo(BlogPost other) { if (equals(other)) {
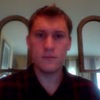
Casey Huckel
Courses Plus Student 4,257 PointsCan someone explain this better? Like Matthew, I'm also stuck

Muhammad Ismail
6,539 PointsAs Dan has explained, you need to specify type with generics, using bracket angle. So the first change will be like;
public class BlogPost implements Comparable<BlogPost>, Serializable {
Now for the compareTo method, you get rid of the downcast by implementing a type of BlogPost
public int compareTo(BlogPost other) {
PS: This is my first Forum post, excuse me if I break some rules.

k doshi
128 PointsWhy not public int compareTo<BlogPost other>

tristan macdonald
1,104 Pointspackage com.example;
import java.io.Serializable; import java.util.Date;
public class BlogPost implements Comparable<BlogPost>, Serializable { private String mAuthor; private String mTitle; private String mBody; private String mCategory; private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) { mAuthor = author; mTitle = title; mBody = body; mCategory = category; mCreationDate = creationDate; }
public int compareTo(BlogPost other) { if (equals(other)) { return 0; } return mCreationDate.compareTo(other.mCreationDate); }
public String[] getWords() { return mBody.split("[^\w']+"); }
public String getAuthor() { return mAuthor; }
public String getTitle() { return mTitle; }
public String getBody() { return mBody; }
public String getCategory() { return mCategory; }
public Date getCreationDate() { return mCreationDate; } }

tristan macdonald
1,104 Pointsthis should help

Imran Asif
7,514 PointsThe first thing we need to make interface Comparable generic. To make it generic : Comparable<T> Comparable interface is implemented by class BlogPost here, so we will use Type variable(Object) BlogPost of class BlogPost. So, we will use Comparable<BlogPost> \ Here BlogPost is Object or Type variable of Class BlogPost
Then in compareTo Method:
public int compareTo(Object obj) { BlogPost other = (BlogPost) obj;
we need to change Object with BlogPost and need to remove type casting:
public int compareTo(BlogPost blogPost) { BlogPost other = blogPost;

k doshi
128 PointsWhy not public int compareTo<BlogPost other>
Casey Huckel
Courses Plus Student 4,257 PointsCasey Huckel
Courses Plus Student 4,257 PointsCan you write out the whole code? I don't know where to put your one line of code.