Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial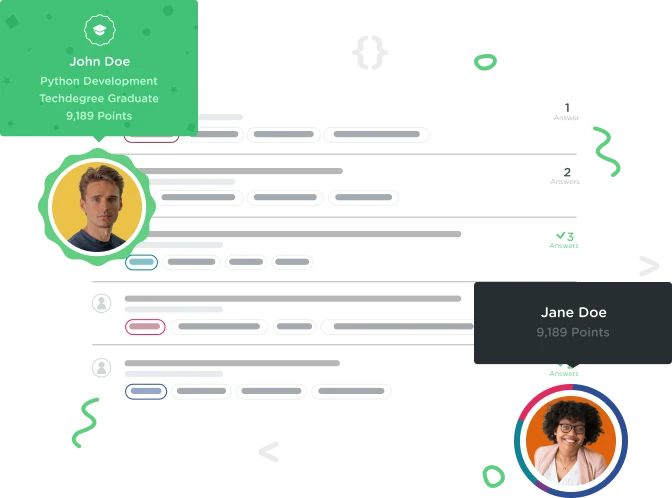
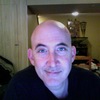
Charles Harpke
33,986 PointsNow we can do something with the item that was tapped on. We have a helper method, sendVote(), that will log the user's
The helper method sendVote is supposed to log the user's vote for their favorite game...Am I calling a 'send vote()' in the item click listener?
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.view.ListView;
public class GameListActivity extends ListActivity {
public String[] mGames = {
"Age of Empires",
"Civilization",
"Minecraft",
"SimCity"
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game_list);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, mGames);
setListAdapter(adapter);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
// Add code here!
super.onListItemClick(l, v, position, id);
}
// This is a helper method to tally votes on a back-end system
public void sendVote(String game) {
VoteService.recordVote(game);
}
}
```java
```GameListActivity.java
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.view.ListView;
public class GameListActivity extends ListActivity {
public String[] mGames = {
"Age of Empires",
"Civilization",
"Minecraft",
"SimCity"
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_game_list);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, mGames);
setListAdapter(adapter);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
// Add code here!
super.onListItemClick(l, v, position, id);
}
// This is a helper method to tally votes on a back-end system
public void sendVote(String game) {
VoteService.recordVote(game);
}
}
1 Answer
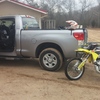
Ryan Ruscett
23,309 PointsHola,
In short yes you are correct. You need to call the sendVote() method inside the on list item click. BUTTT! There is always a but huh.
You need to pass it the proper game from the String Array mGames to the sendVote method.
So first we have the game list
public String[] mGames = {
"Age of Empires",
"Civilization",
"Minecraft",
"SimCity"
};
Ok so check it out. Below is the method for the onListItemClick. We are getting a listView ok fine, but we don't care about that. I mean, I can't get a game from my list with a LIstView I can only display the games. Ok, next we have View v aahhh naaa that is again, just another view. Next we have int position OHHHH ok that sounds pretty good. Let's double check. What comes next? A long... Huh, I can't get a game from a string array with a long. So it looks like position it is. I can get a value from a string array based on it's index. Which is an integer.
protected void onListItemClick(ListView l, View v, int position, long id) {
// Add code here!
super.onListItemClick(l, v, position, id);
String gameValue = mGames[position];
Ok, so for the good part.
I need to take my position, use that to grab whatever game is in the array at that position I clicked from the listVIew. Return it to a variable, and call sendVote(passInTheGameIGotByUsingPosition)
protected void onListItemClick(ListView l, View v, int position, long id) {
// Add code here!
super.onListItemClick(l, v, position, id);
String gameValue = mGames[position];
sendVote(gameValue);
Does this help answer you question? If not please let me know and I can try to explain it, a different way.