Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial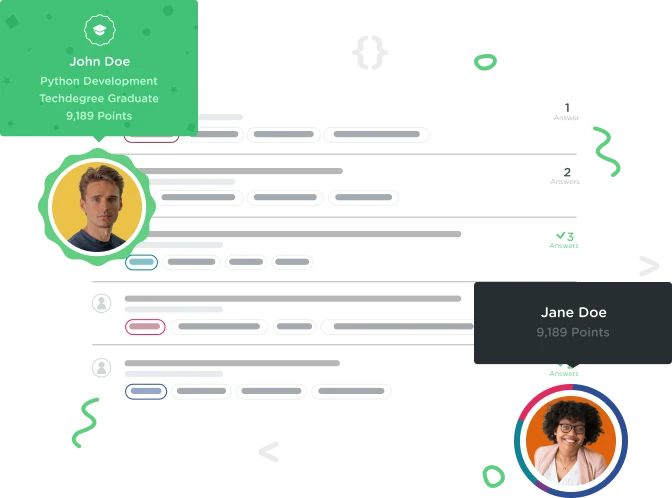
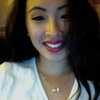
Jennifer Xu
1,716 PointsNSDictionary Part in Blog Reader App Problem, Not Running Code
I've followed everything in the 'What is NSDictionary?' video in configuring my code, but it will not display the text in the cells. I wrote an NSLog line in between the cell.textlabel etc lines, and the console doesn't even run the line, thus it means that it's not reaching that part of code when running! How do I fix this?? Here's my code:
#import "TableViewController.h"
@interface TableViewController ()
@end
@implementation TableViewController
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
NSDictionary *blogPost1 = [NSDictionary dictionaryWithObjectsAndKeys:@"The Missing Widget in Android", @"title", @"Ben Jakuben", @"author", nil];
NSDictionary *blogPost2 = [NSDictionary dictionaryWithObjectsAndKeys:@"Getting started with iOS Development", @"title", @"Amit Bijlani", @"author", nil];
NSDictionary *blogPost3 = [NSDictionary dictionaryWithObjectsAndKeys:@"An Interview with Shay Howe", @"title", @"Joe Villanueva", @"author", nil];
//self.blogPosts = [NSARRay initialize];
self.blogPosts = [NSArray arrayWithObjects:blogPost1, blogPost2, blogPost3, nil];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 0;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
//NSLog(@"preparing for segue: %d",[self.blogPosts count]);
return [self.blogPosts count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
NSDictionary *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
cell.textLabel.text = [blogPost valueForKey:@"title"];
NSLog (@"title %@", [blogPost valueForKey:@"title"]);
cell.detailTextLabel.text = [blogPost valueForKey:@"author"];
NSLog (@"author %@", [blogPost valueForKey:@"author"]);
return cell;
}
/*
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the specified item to be editable.
return YES;
}
*/
/*
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (editingStyle == UITableViewCellEditingStyleDelete) {
// Delete the row from the data source
[tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationFade];
} else if (editingStyle == UITableViewCellEditingStyleInsert) {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath
{
}
*/
/*
// Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the item to be re-orderable.
return YES;
}
*/
/*
#pragma mark - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
// Get the new view controller using [segue destinationViewController].
// Pass the selected object to the new view controller.
}
*/
@end
2 Answers

Hai Phung
5,079 PointsHi!
Your -numberOfSectionsInTableView: method is currently returning 0. This is just boilerplate code that is generated when you create a subclass of UITableViewController. The method actually defaults to one, so you can just remove the method if you only need one section. However, if you want to set it explicitly, just make sure it returns a value of 1.
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
// Return the number of sections.
return 1;
}
Hope that helps!

Stone Preston
42,016 Pointscan you put a few more NSLogs in the other tableView delegate methods for example:
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
//NSLog(@"preparing for segue: %d",[self.blogPosts count]);
NSLog(@"inside number of rows in section");
return [self.blogPosts count];
}
if that log doesnt show in the console something is going on with the delegate.