Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial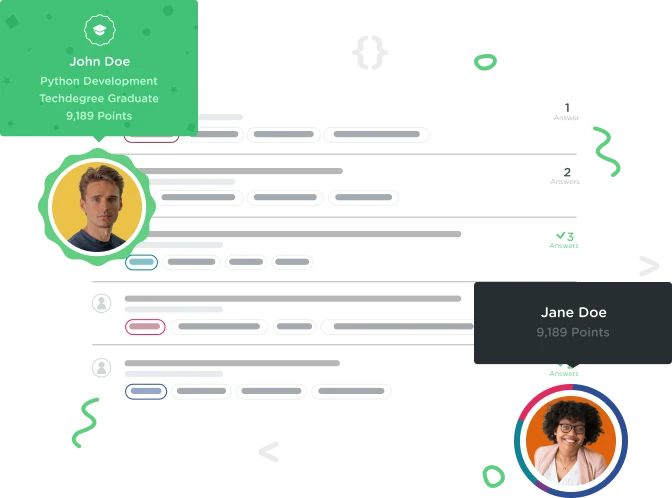
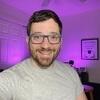
wesley franks
6,198 PointsNull pointer error...
I am actually experiencing the same issue. My code has been written to match the instructor's.
I can show my code here:
---------------------------FunFactsActivity.java--------------------------------------------------------------
package com.megliosolutions.funfacts;
import android.content.DialogInterface;
import android.graphics.Color;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends ActionBarActivity {
private FactBook mFactBook;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our View variables and assign them the views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
final RelativeLayout relativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
// Update the label with our dynamic fact
factLabel.setText("");
relativeLayout.setBackgroundColor(Color.RED);
}
};
showFactButton.setOnClickListener(listener);
}
}
--------------------------------------FactBook.java------------------------------------------------
package com.megliosolutions.funfacts;
import java.util.Random;
/**
* Created by Wesley on 1/8/2015.
*/
public class FactBook {
// Member variable (properties about the object)
public String[] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"Treehouse is not actually in a tree."};
// Method (abilities: these are things the object can do)
public String getFact(){
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); //Construct a new Random number generator
// Assigning an int or number value to choose between
int randomNumber = randomGenerator.nextInt(mFacts.length);
//fact is being made equal to randomNumber adding an empty
fact = randomNumber + "";
fact = mFacts[randomNumber];
return fact;
}
}
I am not sure where my issue is. with the Null pointer error.

Aaron Arkie
5,345 PointsMy code is the following:
package com.arkman.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our view variables and assign them the views from the layout files
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
public void onClick(View view) {
String[] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built." }; // my list of facts held in an Array object
//randomly select a fact
Random randomGenerator = new Random(); // create a reference variable to store the Random class
int randomNumber = randomGenerator.nextInt(mFacts.length); // Create a variable that will be used to store a random int
String fact = ""; // Create a variable that will be used to store a String from my array object named mFacts
fact = mFacts[randomNumber]; // Use the integer stored in randomNumber to hold a position inside my Array Object
//Update the label with our dynamic fact
factLabel.setText(fact); // set my TextView object called factLabel to the String stored in fact
}
};
showFactButton.setOnClickListener(listener); // wait for my Button to be pressed. Then update using the onClick method.
}
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts, menu);
return true;
}
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}

Ken Alger
Treehouse TeacherEdited original post for markup
13 Answers

Aaron Arkie
5,345 PointsYeah like Thomas Edison said "I have not failed. I've just found 10,000 ways that won't work." Keep at it and you're bound to come to a solvable solution. Plus it also helps others who may have questions or the same error and they can look at your steps and follow along to solve it. The Java Object projects are really interesting. If you are new to the language i highly recommend this part of the android track. Craig Dennis really gets into the language and shows you neat methods and programming styles to enhance your code. Moreover, since JAVA is OOP it's best to get the overall grasps of the use of objects as it makes it easier to take advantage of the language.
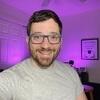
wesley franks
6,198 PointsI FIGURED IT OUT!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
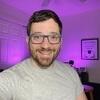
wesley franks
6,198 PointsOk so apparently I missed one last crucial step. One thing is important when watching these videos everyone is don't do it when you could be possibly distracted! LOL. Missed these small tiny little things. Oh well, ok so onto the solutions. In making sure that the above mistakes that I made were fixed in your code, here is where I went wrong and got the NULL pointer error.
in the following code I will demonstrate the error that I didn't see until now.
private FactBook mFactBook;
when I should have paid a bit more attention and added this extra step:
private FactBook mFactBook = new FactBook();
fixed it and now I am onto the next step!!
Hope this helps, thank you everyone for your support! Love this site!! Definitely going to continue to learn knowing that I can get help when needed! Thanks!!!
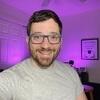
wesley franks
6,198 PointsI am still confused because I have an activity java file, and a class file. I have seperated the code for DRY, and to allow for there not to be so much code in one file when I can make a class to be pointed to.
I guess I am just confused because I have followed the video exactly or so I think. Which is why I posted my code. I guess I am still not understanding what is wrong.
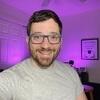
wesley franks
6,198 PointsTake that back! after looking through the video I missed a crucial step that we all let go over our heads! No worries we're a team! Here's where I messed up.
View.OnClickListener listener = new View.OnClickListener() { @Override public void onClick(View v) { String fact = mFactBook.getFact(); // Update the label with our dynamic fact factLabel.setText(fact); // <------------------------- RIGHT HERE ---------------------- // I didn't make the "factLabel.setText(""); back to it's original "...setText(fact); relativeLayout.setBackgroundColor(Color.RED); } }; showFactButton.setOnClickListener(listener);
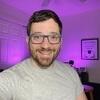
wesley franks
6,198 PointsAh dang, that didn't work. Well I am glad I am at least fixing some things! I will continue to see what I missed! I do these classes on my lunch break and get asked questions from co-workers while listening so I am assuming that is what happened. I just missed a few things!

Aaron Arkie
5,345 PointsHey Wesley! No worries! Once i spent two hours trying to figure out why my code kept returning an error and it was cause i closed an if statement to early. Once you solve it though you feel like a champ! On the other hand i think i had the same error that you were having. Check the exception error and see if it tells you were the error is located. i believe it has to do with the String fact label. If your code is just String fact; <--- at runtime the compiler sets it to null. If you would like to compare on this thread i will leave my code which is identical to the instructors. (of course with some of my own comments).
package com.arkman.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our view variables and assign them the views from the layout files
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
public void onClick(View view) {
String[] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built." }; // my list of facts held in an Array object
//randomly select a fact
Random randomGenerator = new Random(); // create a reference variable to store the Random class
int randomNumber = randomGenerator.nextInt(mFacts.length); // Create a variable that will be used to store a random int
String fact = ""; // Create a variable that will be used to store a String from my array object named mFacts
fact = mFacts[randomNumber]; // Use the integer stored in randomNumber to hold a position inside my Array Object
//Update the label with our dynamic fact
factLabel.setText(fact); // set my TextView object called factLabel to the String stored in fact
}
};
showFactButton.setOnClickListener(listener); // wait for my Button to be pressed. Then update using the onClick method.
}
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts, menu);
return true;
}
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
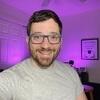
wesley franks
6,198 PointsWell your code shows everything in one file. I have separated my code into a java file and .class file. Also I think this step right here is what I am missing in my class file. But I am not sure. In the video step one of "Dynamically Changing the Background Color". This step is what I am referring to.
"Declare a member variable with the data type of RelativeLayout. The format is just like our other member variables but with this other data type and a different name. "

Aaron Arkie
5,345 PointsOh i see! I think you are ahead of me, i stopped to do the new updated section of Java objects for this track. Once i get here i will see what's going on and ill lay down some more input. Good luck and let me know if you figure it out!
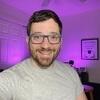
wesley franks
6,198 PointsNo worries! I just can't stop and do something else. My brain just goes nuts haha. So I have to finish this. Once I am done I will do the objects lemme know if it helps better.
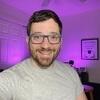
wesley franks
6,198 Pointsmuch appreciated definitely understand and agree. I will never quit. I will definitely find a good way to solve it. Just takes trial and error! I will post here if I find an answer!

Aaron Arkie
5,345 PointsAhh this problem was going through my head too. After much thought i figured you forgot to create a reference object to the class you created. I remember you saying you created a separate class to make it more efficient to read! Great job! told you you'd feel like a champ! Well done!
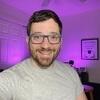
wesley franks
6,198 PointsI thought my answer posted, I guess not.
You were correct Aaron, it was the declaration of the class variable.
I fixed it after staying up for a few extra hours! Much appreciated definitely remembering that for next time! Oh and btw TREEHOUSE I LOVE YOU!
having the ability to do these courses on any platform is amazing!
Aaron Arkie
5,345 PointsAaron Arkie
5,345 PointsOkay I see the issue. Lets break it down
I really hope this helps you! I will leave my code for you to compare it too.