Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial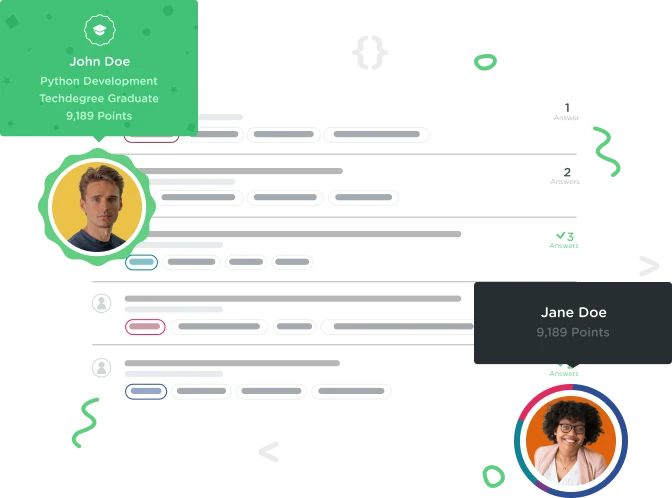
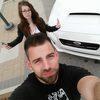
Jordan Ernst
5,121 Pointsnull pointer exception.
i am getting a runtime error of: Null pointer exception line 58 in ListAdapter which is this line
holder.holeLabel.setText(mHoles[position].getLabel());
here is the rest of my code for ListAdapter:
package com.jordanernst.golfscorecard;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.TextView;
/**
* Created by insan_000 on 2016-03-21.
*/
public class ListAdapter extends BaseAdapter {
private final Hole[] mHoles;
private final Context mContext;
public ListAdapter(Context context, Hole[] holes){
mContext= context;
mHoles = holes;
}
@Override
public int getCount() {
return mHoles.length;
}
@Override
public Object getItem(int position) {
return mHoles[position];
}
@Override
public long getItemId(int position) {
return 0;// not implemented
}
@Override
public View getView(final int position, View convertView, ViewGroup parent) {
final ViewHolder holder;
if(convertView == null){
convertView= LayoutInflater.from(mContext).inflate(R.layout.list_item, null);// ibelieve passing null here is wrong
holder= new ViewHolder();
holder.holeLabel = (TextView) convertView.findViewById(R.id.holeLabel);
holder.strokeCount = (TextView) convertView.findViewById(R.id.strokeCount);
holder.removeStrokeButton = (Button) convertView.findViewById(R.id.removeStrokeButton);
holder.addStrokeButton = (Button) convertView.findViewById(R.id.addStrokeButton);
convertView.setTag(holder);
}
else{
holder = (ViewHolder) convertView.getTag();
}
holder.holeLabel.setText(mHoles[position].getLabel());
holder.strokeCount.setText(mHoles[position].getStrokeCount());
holder.removeStrokeButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int updatedStrokeCount = mHoles[position].getStrokeCount() - 1;
if (updatedStrokeCount < 0) updatedStrokeCount = 0;
mHoles[position].setStrokeCount(updatedStrokeCount);
holder.strokeCount.setText(updatedStrokeCount + "");
}
});
holder.addStrokeButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int updatedStrokeCount = mHoles[position].getStrokeCount() + 1;
mHoles[position].setStrokeCount(updatedStrokeCount);
holder.strokeCount.setText(updatedStrokeCount + "");
}
});
return convertView;
}
private static class ViewHolder{
TextView holeLabel;
TextView strokeCount;
Button addStrokeButton;
Button removeStrokeButton;
}
}
1 Answer

vicente lee
6,271 PointsChange this:
holder.strokeCount.setText(mHoles[position].getStrokeCount());
To this:
holder.strokeCount.setText(mHoles[position].getStrokeCount() + "");
This setText() expects a String but you're giving it an int.
Jordan Ernst
5,121 PointsJordan Ernst
5,121 Pointsso it appears that mHoles list is null and so is the position