Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial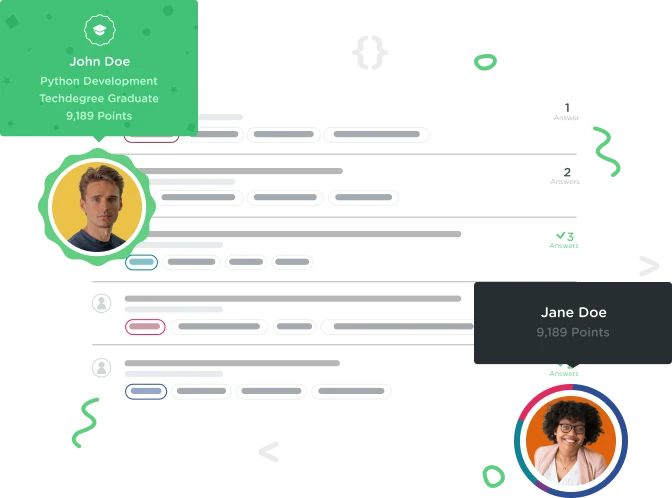

Ganta Hemanth
6,460 PointsNull pointer exception in java
I am getting the following error in the code
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference.
at com.example.hemanth.stromy.MainActivity.updateDisplay(MainActivity.java:96)
at com.example.hemanth.stromy.MainActivity.access$200(MainActivity.java:31)
at com.example.hemanth.stromy.MainActivity$1$1.run(MainActivity.java:72)
package com.example.hemanth.stromy;
import android.app.AlertDialog;
import android.app.DownloadManager;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import butterknife.InjectView;
public class MainActivity extends ActionBarActivity {
private CurrentWeather mcurrentweather;
public static final String TAG = MainActivity.class.getSimpleName();
@InjectView(R.id.temperatureLabel)TextView mTemperatureLabel;
@InjectView(R.id.humidityValue) TextView mHumiditylable;
@InjectView(R.id.precipValue) TextView mPreciplable;
@InjectView(R.id.summaryLabel) TextView mSummaryLabel;
@InjectView(R.id.timelabel)TextView mTimeLabel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "2b345aa7cb761cc607bce49e23e4cb72";
double latitude = 37.8267;
double longitude = -122.423;
String apiUrl = "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+","+longitude;
if(isNetworkAvailable()) {
OkHttpClient Client = new OkHttpClient();
Request request = new Request.Builder().url(apiUrl).build();
Call call = Client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
Toast.makeText(MainActivity.this,"There are network problems",Toast.LENGTH_LONG);
}
@Override
public void onResponse(Response response) {
try {
String jasonData = response.body().string();
if (response.isSuccessful()) {
Log.v(TAG,jasonData);
mcurrentweather = getCurrentDetails(jasonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUser();
}
}
catch (IOException e) {
Log.e(TAG, "expection is ", e);
}
catch (JSONException e){
Log.e(TAG, "expection is ", e);
}
}
});
}
else{
Toast.makeText(this,"The network is unavailable",Toast.LENGTH_LONG).show();
}
}
private void updateDisplay() {
mTemperatureLabel.setText(mcurrentweather.getmTemeperature()+"");
mTimeLabel.setText("At "+mcurrentweather.getFormattedString()+" it will be ");
mHumiditylable.setText(mcurrentweather.getmHumidity()+"");
mPreciplable.setText(mcurrentweather.getmPrecipChance() + "");
mSummaryLabel.setText(mcurrentweather.getmSummary());
}
private CurrentWeather getCurrentDetails(String jasonData) throws JSONException{
JSONObject forecast = new JSONObject(jasonData);
String timezone = forecast.getString("timezone");
JSONObject current = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setmHumidity(current.getDouble("humidity"));
currentWeather.setmTemeperature(current.getDouble("temperature"));
currentWeather.setmTimeZone(timezone);
currentWeather.setMtime(current.getInt("time"));
Log.i(TAG,"From Json : "+timezone);
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUser() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(),"Error_dialog");
}
}
5 Answers

Jason Laux
3,128 PointsHi Ganta,
I had the same problem as you: the was no mTemperatureLabel variable to set text on. I found out that I had forgotten to include the following line of code after the setContentView method call in the onCreate method definition:
ButterKnife.inject(this);
Looking at your onCreate method, I didn't see this line. If your solution was different, please post it.
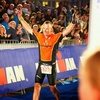
Steve Hunter
57,712 PointsHi Ganta,
I'd recommend you have a read through the following post that is tracking the same problem.
There's a suggestion as to how to tackle debugging the code; give that a try and get back to me with the result.
We can get to the bottom of it shortly!
Steve.

Ganta Hemanth
6,460 PointsTHanks for the quick reply..
I debugged the code. in the line
mTemperatureLabel.setText(mcurrentweather.getmTemeperature()+"");
mTemperatureLabel value is null. The program is being crashed as the null value is calling the setText().
How to fix it??
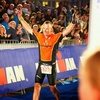
Steve Hunter
57,712 PointsHi Ganta,
Yes, that is where the error is showing up - that's the bit that causing the fatal error due to a null pointer. The problem isn't there, though.
Have a go at this:
OK - so something is null ... I suggest you add a breakpoint at the previous line to the error which, in my code, is: Day day = mDays[position];
. Then run the code using the debugger, not just the normal play button. This will take a little time to get going but be patient!
The code should execute and you can get to the breakpoint by hitting the 7-DAYS button. The bottom of your Android Studio screen should then fill with info.
You should see some variables listed in the central lower pane. One of these should be this, which is expandable. Inside of this you should have mDays which is also expandable. Does it contain any data? You should see your mIcon, mSummary, mTimezone
etc. inside all of the 8 items that mDays[]
should contain.
Click the Step-Over button to move execution to the next line. You shold now have the instance of Day
that you created called day which is expandable. Does it contain sensible data? Further, you should see holder.iconImageView
too - does that expand to show sensible info?
Try all that as that brings us nearer to the actual problem, i.e. the cause, not the symptom which is what your error is. We need to get to the bottom of what is null
that shouldn't be. That error is only part of the story.
Steve.

Emile conde
1,877 PointsHey I am having the same problem. Did you solve yours? If so, how? Thanks.
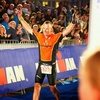
Steve Hunter
57,712 PointsHi Emile,
Have you tried all the suggestions in this thread, especially the one marked as 'Best Answer'?
If so, then we need to see what your Logcat is showing - is it precisely the same line that causing the problem?
Steve.

Emile conde
1,877 PointsYes we have the same LogCat. Our problems are identical.
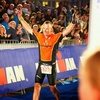
Steve Hunter
57,712 PointsThen the answer is in the thread, right?
Steve.

Emile conde
1,877 PointsThe first answer didn't work for me. I'll try your suggestion later. Thanks for the fast replies.
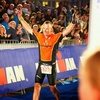
Steve Hunter
57,712 PointsCool - give them a go first as they'd be my starting point if we were creating this thread from scratch. If that doesn't work, then post your code and logcat back here and we can go from there. If you use Github, you can upload you code into that so I can pull it down to my machine and try the debugging myself.
Make sense?
Steve.