Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial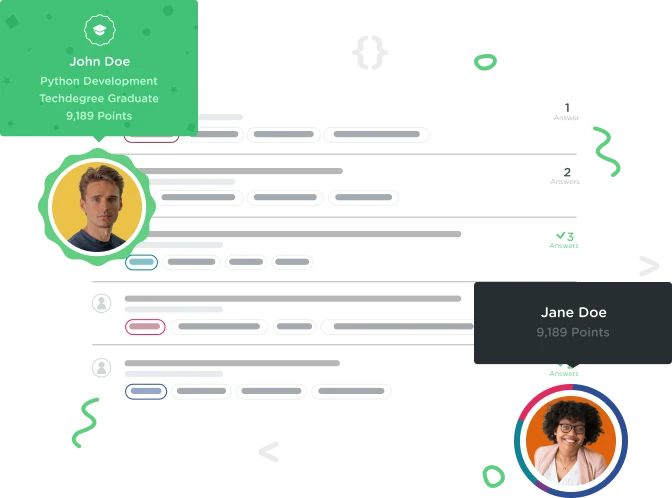

Fahman Khan
3,798 PointsNullPointer Exception Error
Error:(52, 23) error: exception IOException is never thrown in body of corresponding try statement.
I'm not understand how to resolve this issue. I have written the code exactly the way teamtreehouse, but not sure how to fix this. Any solution to this would be appreciated.
The error is in this piece of code as it was underlined red
"catch(IOException e)"
Here is my full code.
package fahmanenterprisesinc.stormy;
import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.util.Log;
import com.squareup.okhttp.Call; import com.squareup.okhttp.Callback; import com.squareup.okhttp.OkHttpClient; import com.squareup.okhttp.Request; import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends ActionBarActivity { private static final String TAG=MainActivity.class.getClass().getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "cb7e86df34750d1748b5e82ce52b87dc";
double latitude = 37.8267;
double longitude = -122.423;
//Create a url for the website
String url = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + "," + longitude;
OkHttpClient myClient = new OkHttpClient();
Request myRequest = new Request.Builder().url(url).build();
//Put the request inside the call object
Call myCall = myClient.newCall(myRequest);
//Execute the code
myCall.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException
{
try{
if(response.isSuccessful())
{
Log.v(TAG, response.body().toString());
}
System.out.print(response.toString());
} catch(IOException e)
{
Log.e(TAG, "EXCEPTION ERROR", e);
}
}
});
}
}
2 Answers

James Simshaw
28,738 PointsHello,
I'm not sure about any NullPointerExceptions in your code, but I can tell you why Android Studio is complaining about your catch(IOException e) line. None of the code in
try{
if(response.isSuccessful())
{
Log.v(TAG, response.body().toString());
}
System.out.print(response.toString());
}
will throw an IOException, so its complaining about an exception attempting to be caught that cannot ever be thrown. The reason Ben has the try catch block is that he does something slightly different than you are. Instead of
//Execute the code
myCall.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException
{
try{
if(response.isSuccessful())
{
Log.v(TAG, response.body().toString());
}
System.out.print(response.toString());
} catch(IOException e)
{
Log.e(TAG, "EXCEPTION ERROR", e);
}
}
});
He calls
try{
Response response = myCall.execute();
if(response.isSuccessful()) {
Log.v(TAG, response.body().toString());
}
} catch(IOException e) {
Log.e(TAG, "EXCEPTION ERROR", e);
}
In which the myCall.execute() can throw an IOException so he has to deal with it(which he does by catching and handling the Exception). Please let us know if this helps and if you need more assistance so we can assist you further. Also, if you need more assistance, please provide your updated code so we can help you from your current setup.

Fahman Khan
3,798 PointsSweet!! That works. Thank you very much.