Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial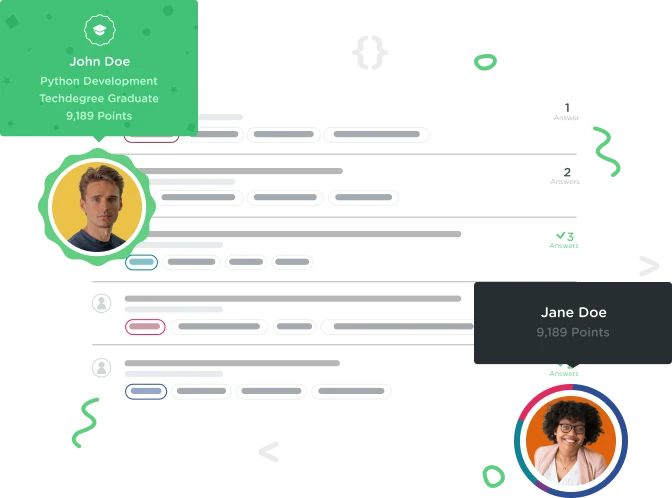

Joseph Anderson
10,617 PointsNullPointerException in Code Challenge
First off, having the escape key near the ` key is dangerous. This is my second time trying to write this question...
Anyway, I'm getting a NullPointerException once as I try to place the csr back into the queue.
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
while (mSupportReps == null) {
playHoldMusic();
}
csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
}
Everything is fine and dandy until I add the final line containing the add() method--up until then the error message is "Did you forget to add the rep back into the queue?". I'm not sure if it's telling me my csr is null, my queue is null or what? I'm guessing I've failed to initalize something somewhere? Thanks in advance.

Joseph Anderson
10,617 PointsIt tells me the error is on the assist method but interestingly enough I get no error if I compile the code without the final add method, I just get reminded that i need to return the CustomerServiceRep to the queue.

Joseph Anderson
10,617 PointsThe plot thickens a bit as this configuration (as above):
csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
throws:
java.lang.NullPointerException
at CallCenter.acceptCustomer(CallCenter.java:29)
at JavaTester.run(JavaTester.java:110)
at JavaTester.main(JavaTester.java:41)
but if i order the methods differently:
csr = mSupportReps.poll();
mSupportReps.add(csr);
csr.assist(customer);
I get a crazy:
java.lang.NullPointerException
at java.util.ArrayDeque.addLast(ArrayDeque.java:244)
at java.util.ArrayDeque.add(ArrayDeque.java:418)
at CallCenter.acceptCustomer(CallCenter.java:29)
at JavaTester.run(JavaTester.java:110)
at JavaTester.main(JavaTester.java:41)
To wit, the assist() method looks like this:
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
1 Answer
Christopher Augg
21,223 PointsJoseph,
Actually, your code is almost perfect! You only have one mistake and it is in your while loop. Lets get this working for you:
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
// you are testing if the memory reference of the queue is null here.
// You need to test if an element within the queue is null by using .peek()
while (mSupportReps == null) {
playHoldMusic();
}
csr = mSupportReps.poll();
csr.assist(customer);
mSupportReps.add(csr);
}
Basically, you test if you have a reference to the queue and since you do the loop never happens. Therefore, you attempt to assign null to car because nothing is in the queue yet. By using .peek() you can check if something is in the queue without removing it from the queue like .poll() does.
Hope this helps.
Regards,
Chris

Joseph Anderson
10,617 PointsThank you Chris. Certainly was forced to give myself an education on NullPointerExceptions, ha. Thanks for pointing out .peek() too.
Enrique Munguía
14,311 PointsEnrique Munguía
14,311 PointsCan you provide the stack trace?, usually it gives the exact location of the error.