Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial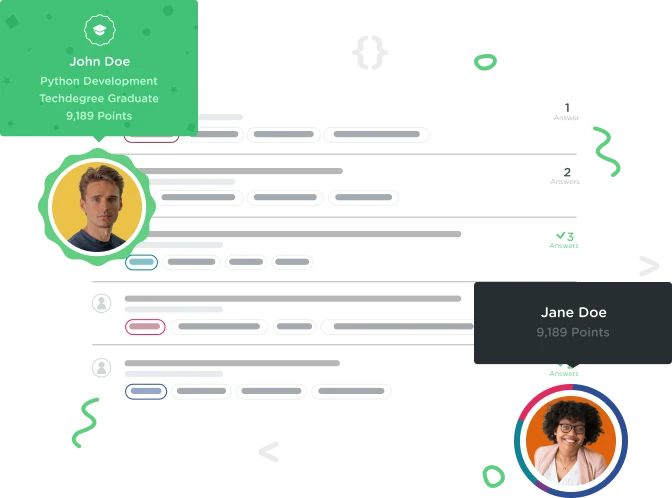

Bruce Parmele
6,474 PointsNullPointerException when using EditText getText().toString()...
While debugging an issue I am having, as soon as I step over to this line, "String username = mUsername.getText().toString();" in the onlciklistnener of the SignUpActivity class, I get a NullPointerException. It will throw this error with any of the variables that use .getText().toString().
I am lost. Any help is appreciated
5 Answers

Bruce Parmele
6,474 PointsOk - This is what I was doing wrong. I was referencing the field in activity_login and not activity_sign_up. All is good with the world now :)

miguelcastro2
Courses Plus Student 6,573 PointsIf the field has no value present it will be set to NULL and if you try to run the toString() method you will receive this error. Check for null prior to retrieving the value:
if (mUsername.getText() != null) {
myUsernameStr = mUsername.getText().toString();
}
Jonathan Lay
8,468 Points(See my longer Answer for an explanation of how I solved it, with my code snippet.)
In my case, this did not solve the problem but it added a new problem. The new problem: just the condition clause in the if statement caused a null pointer error to be raised. You can work around the new problem by checking mUsername for null without invoking getText(), like this: String username = ""; if (mUsername != null){username = mUsername.getText().toString();}
But the underlying problem is that there are 2 pair of EditText items with the same name, one pair in activity_login.xml and the other in activity_sign_up.xml. When you access the value by using mUsername = (EditText)findViewById(R.id.usernameField); it is ambiguous which EditText field you are accessing. If you access the wrong one, you will get a null value instead of a string.

Bruce Parmele
6,474 PointsThanks Miguel. The code I am using is directly from Ben's video(and has no null check) and his code doesn't generate the errors. I will use the null check you suggested so I can move on.
Thanks again!

Bruce Parmele
6,474 PointsI've tried the null check and it only added more problems. I would like the code to execute just like it does in Ben's video without modification. I am using eclipse Luna. AS you can see it is the same code as in the video.
mSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
String email = mEmail.getText().toString();
username = username.trim();
password = password.trim();
email = email.trim();
if (username.isEmpty() || password.isEmpty() || email.isEmpty()) {
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.signup_error_message)
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
// create the new user!
}
}
});
}
Jonathan Lay
8,468 PointsI am using Android Studio as the IDE. I solved the problem by assigning different IDs to the EditText fields in activity_login and activity_sign_up. The video shows naming the EditText fields in both activities with the same names: usernameField and passwordField. The problem with this is when you access them later using (for example) mUsername = (EditText)findViewById(R.id.usernameField); it is ambiguous which activity you will be accessing the usernameField in. So, I gave the fields unique IDs: signupUsernameField, signupPasswordField, loginUsernameField, and loginPasswordField. After doing that, the code below works.
mUsername = (EditText)findViewById(R.id.signupUsernameField);
mPassword = (EditText)findViewById(R.id.signupPasswordField);
mEmail = (EditText)findViewById(R.id.signupEmailField);
mSignUpButton = (Button)findViewById(R.id.signupButton);
mSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString();
String password = mPassword.getText().toString();
String email = mEmail.getText().toString();
username = username.trim();
password = password.trim();
email = email.trim();
if (username.isEmpty() || password.isEmpty() || email.isEmpty()){
AlertDialog.Builder builder = new AlertDialog.Builder(SignUpActivity.this);
builder.setMessage(R.string.signup_error_message)
.setTitle(R.string.signup_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
// create the new user