Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial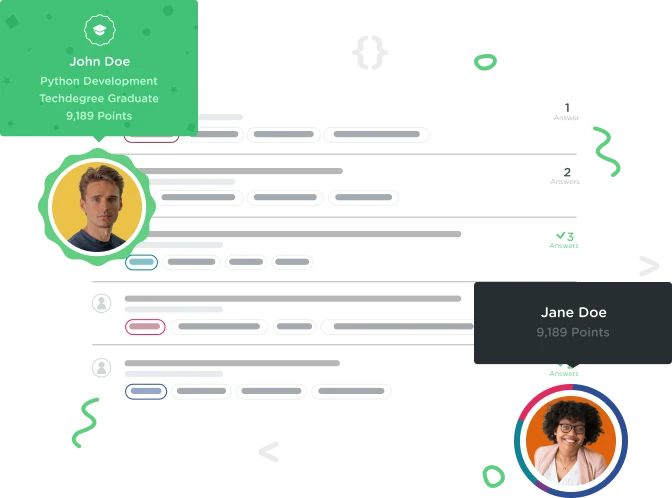

Lauren Gainsbrook
12,539 PointsNumber game - no numbers left for computer to select
I'm working on the challenge after the number game task (do the reverse, where the computer tries to guess the number that a user has chosen). It's working fine, except if the user makes a mistake and it somehow ends up that there are no more numbers for the computer to choose from. For example:
- computer guesses 31, user says "too high"
- computer guesses 30, user says "too low"
- the computer now has no more numbers to guess, and the program just stops. How can I print a message/have the program restart in this scenario?
def game():
# Get range from user and give instructions.
print "First, think of a secret number between 1 and 10, 100, or 1,000."
upper_limit = int(raw_input("Is the upper limit of your number 10, 100, or 1,000?\n"))
lower_limit = 1
print """
Thank you! Now the computer will try to guess your secret number. After each guess, you will be prompted
to tell it if the guess is 'too high', 'too low', or 'correct'.
"""
import random
all_guesses = []
while True:
guess = random.randint(lower_limit, upper_limit)
if not guess in all_guesses:
all_guesses.append(guess)
print "The computer's guess is: {}.".format(guess)
feedback = raw_input("Enter 'too high', 'too low', or 'correct'\n")
if feedback == 'too high':
upper_limit = guess
elif feedback == 'too low':
lower_limit = guess
elif feedback == 'correct':
break
else:
continue
game()
print "Game over. Thanks for playing!"
2 Answers
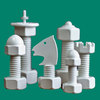
Steven Parker
231,533 PointsYou could test for that condition at the beginning of the loop:
if lower_limit >= upper_limit - 1:
print "It looks like you gave me some bad feedback! I guess we should start over."
break
If you want to start over instead of ending, you could replace break
with game()
. But then later when you really do want to end you'd need to replace the other break
with exit()
Also, it looks like you're still using Python version 2. Time to upgrade?
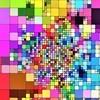
james south
Front End Web Development Techdegree Graduate 33,271 Pointsin your scenario the program enters an infinite loop because there are no more random integers between lower and upper that haven't already been chosen and appended to the list, so the loop runs, the conditional statement is false (number is already in the all guesses list), and so the continue statement at the bottom is hit, and on and on forever. you need to check that the difference between lower and upper isn't 1, and if so, break.