Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial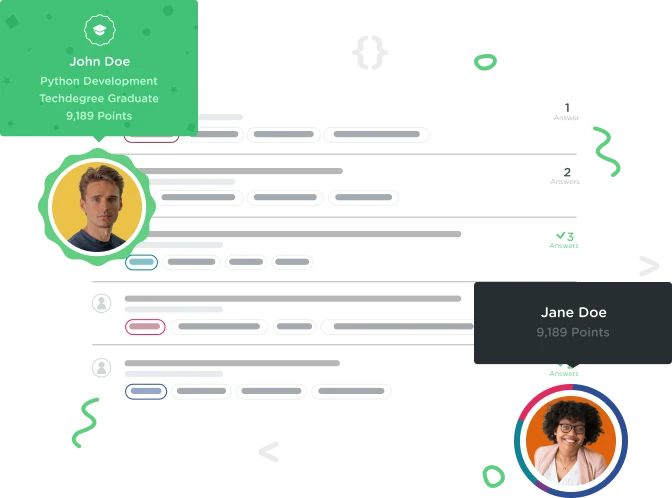

Mohamed THIAM
1,091 PointsNumber Game Refinement.
Hello everyone,
So I've managed to do most of the challenge on my own but I'm having certain issues that I can't seem to solve on my own.
1 - If my first input isn't an int, I get an error.
2 - When I make my 5 mistakes, my code will print whether the input is higher/lower than the secret number instead of going straight to: print('That wasn't it.. My number was {}'.format(secret_num))
Also please give me feedback on the cleanliness of the code or any other advice or comment that could help.
Cheers,
Mo.
import random
def num_game():
secret_num = random.randint(1, 25)
attempt = []
print('Welcome to the number game! You only have 7 attempts!')
print('Guess a number that\'s between 1 and 25:')
while len(attempt) < 7:
try:
guess = int(input())
except ValueError:
print('{} is not a number!'.format(guess))
else:
if guess == secret_num:
print('Congratulations! That\'s it! You\'ve found my number!')
break
elif guess < secret_num:
print('You\'re number is higher than {}!'.format(guess))
else:
guess > secret_num
print('You\'re number is lower than {}!'.format(guess))
attempt.append(guess)
else:
print('That wasn\'t it.. My number was {}!'.format(secret_num))
play_again = input('Do you want to play again? Y/N')
if play_again == 'Y':
print('Cool!')
num_game()
else:
print('Got it. See you next time!')
num_game()
1 Answer
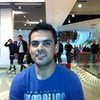
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Mohamed
I did this game too, but didnt realise the bug regards the trying to convert the input to an integer, it looks like when it hits that ValueError the variable guess doesnt get assigned, hence when you try and print it out it goes all crazy. In regrads to your program exiting at 5 tries the reason for this is you have set the length of attempt to less than 7,
change the following lines of code and it should work.
while len(attempt) < 5:
try:
guess = int(input())
except ValueError:
print('Only whole numbers are allowed !)