Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial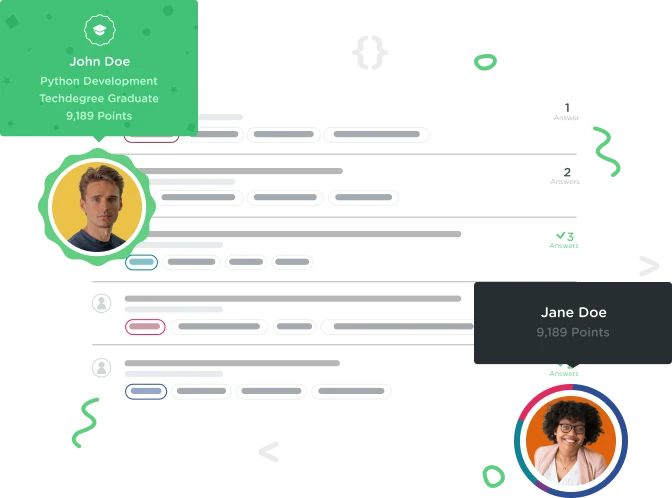

Rob Grieves
4,898 PointsNumber Game Refinement issue: UnboundLocalError: local variable 'guess' referenced before assignment
When I enter a ValueError for my guess the programme spags out and gives me an error. I can't seem to find what the issue is. Can anyone help please?
This is my code:
import random
def game(): # generate a random number between 1 - 10 secret_num = random.randint(1, 10) guesses = []
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a random number between 1 and 10. You have 5 attempts: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}.".format(guess))
else:
print("My number is lower than that.".format(guess))
guesses.append(guess)
else:
print("You didn't get it! My secret number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()
The error I'm given is:
Guess a random number between 1 and 10. You have 5 attempts: f
Traceback (most recent call last):
File "number_game.py", line 11, in game
guess = int(input("Guess a random number between 1 and 10. You have 5 attempts: "))
ValueError: invalid literal for int() with base 10: 'f'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "number_game.py", line 32, in <module>
game()
File "number_game.py", line 13, in game
print("{} isn't a number!".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
1 Answer
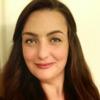
Jennifer Nordell
Treehouse TeacherHi there! This bug is absolutely intentional. In fact, they mention it in the Teacher's Notes. But I can explain what's happening. You are trying to assign an int to guess, but when that fails (ie you entered the letter "f"), the assignment never occurs. In your error handling, you then try and format the string with the value of guess, but again, that assignment never happened. It failed.
Take a look at the Teacher's Notes
Hope this helps!
Rob Grieves
4,898 PointsRob Grieves
4,898 PointsAh thank you, that makes sense! I'll make sure to check the Teacher's Notes in future.
Ikechukwu Arum
5,337 PointsIkechukwu Arum
5,337 PointsThe teacher's note is still not clear in what we should do. How do you take out the string formatting?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherIkechukwu Arum I'd do it with something like this:
print("That isn't a number.")
. In my opinion, it's not needed to tell them what they typed that wasn't a number. They can already clearly see it on their screen.Alternatively, if you really want to use the string formatting, you'd have to move the prompt for the guess outside of the try at the top and then do
guess = int(guess)
inside thetry
. This would allow you to leave the formatting in place, but again, I really feel like it's overkill.