Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial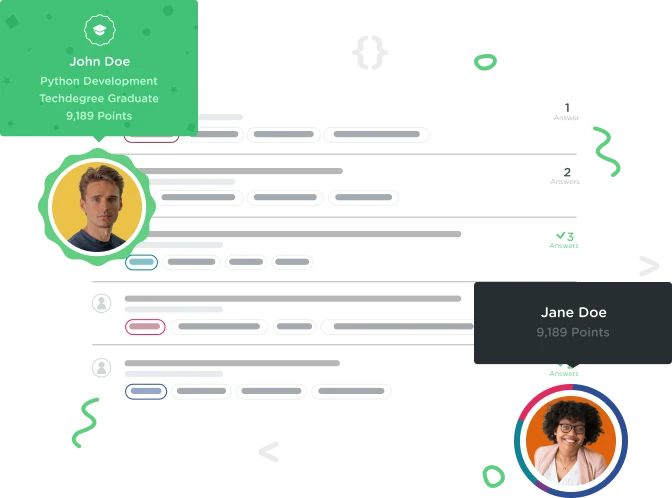
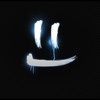
Grigorij Schleifer
10,365 PointsNumber guess game "reversed"
Hey treehouse friends,
I nee help understanding the variable concept inside python.
I have created a number game where the computer guesses the number of the user.
But it doesnt work ;((((
# -*- coding: utf-8 -*-
import random
# create a list to store the computer guesses
list = []
print("Input a number between 0 and 10")
# user input method
def input_number():
while True:
try:
num_to_guess = int(raw_input("> "))
break
except ValueError:
print("Something went wrong")
def game():
# let the computer gueess until the length of the list is 10
while len(list) < 10:
# create the random number
pc_num = random.randint(0, 10)
list.append(pc_num)
if pc_num == num_to_guess:
print("PC got it")
break
#call the method to get the number from the user
input_number()
# start the game
game()
#print the list
print(list)
The error is:
Input a number between 0 and 10
> 6
Traceback (most recent call last):
File "reverse_guess_game.py", line 34, in <module>
game()
File "reverse_guess_game.py", line 26, in game
if pc_num == num_to_guess:
NameError: global name 'num_to_guess' is not defined
What is the meaning of the global name not defined?
Every help is highly appreciated.
Grigorij
1 Answer
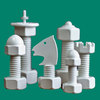
Steven Parker
231,275 PointsYour issue seems to be because num_to_guess is defined within the input_number() function, but the game() function is trying to access it.
try adding
num_to_guess = 0
up where you define list. That will make it global, and accessible by both functions.
Actually, the first error I got when trying this was "NameError: name 'raw_input' is not defined". Is there a missing import? But I changed it to plain input.
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey Steven,
thank you, I got it.