Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial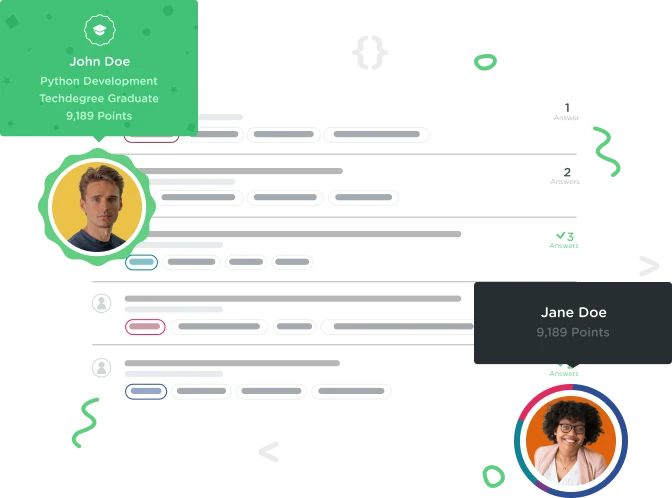
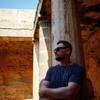
mersadajan
21,306 PointsNumber of characters excluding spaces
I thought we had to count all characters in the string excluding all spaces and wrote this code:
const myString = {
string: "Programming with Treehouse is fun!",
countWords: function () {
const words = this.string.split(" ");
return words.length;
}
}
myString.countWords();
var numWords = myString.countWords();
myString.characters = function() {
const character = this.string.replace(/\s+/g,"");
return character.length;
}
console.log(myString.characters());
I was stuck on this challenge for a long time :( The task was too confusing as it was too simple and unnecessary. Why would I save the length of a string in a property if that string has it*s own length property? And are spaces considered characters?
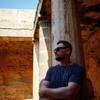
mersadajan
21,306 PointsThank you Rich Donnellan! I was wondering what happened haha

Michael Macdonald
34,265 PointsYeah, I agree with mersadajan. The explanation for the task was kinda confusing.. I must have chewed up half an hour looking for at regex for JavaScript. Which, I haven't covered yet in JavaScipt, but luckily I learnt it from Craig through Java. Then only to find, that they wanted the length...lol Gotta remember some of us ain't that smart... I failed highschool english 2x, then dropped out, lucky I have google, lol
2 Answers
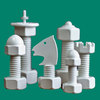
Steven Parker
231,275 PointsYou were getting way too fancy there! The challenge doesn't ask for a method, just a property containing a value.
And yes, spaces are included in the characters. And you're also right that this would not be terribly useful since you can always get the length of the string property directly. But since the point of the challenge was just to practice creating new properties, it served that purpose.

Paul May
3,432 PointsI agree the challenge was a little ambiguous. I think an easier way to accomplish the same result without having to manipulate the string with regex would be something like
const numCharacters = myString.string.length - myString.numWords;
Since we defined the numWords variable in the last challenge which holds the number of spaces, we can simply take this from that value from the length of the string and be left with the number of characters.
Rich Donnellan
Treehouse Moderator 27,708 PointsRich Donnellan
Treehouse Moderator 27,708 PointsQuestion updated with code formatting. Check out the Markdown Cheatsheet below the Add an Answer submission for syntax examples.