Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial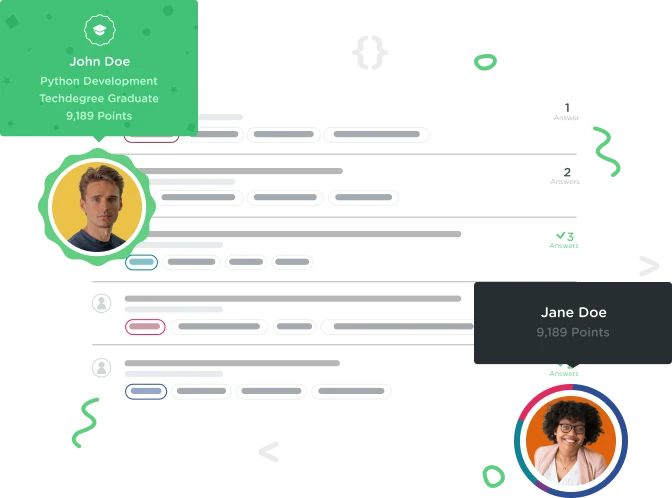

tyler bucasas
2,453 Pointsnumbers_game
import random
def game(): # generate a random number from 1 to 10 secret_num = random.randint(1, 10) guesses = []
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("you got it! my number was {}".format(secret_num))
break
elif guess < secret_num:
print("my number is greater than {}".format(guess))
else:
print("my number is less than {}".format(guess))
guesses.append(guess)
else:
print("you didnt get it my number was {}".format(secret_num))
play_again = input ("do you want to play again Y/n")
if play_again.lower() != 'n':
game()
else:
print("peace out ")
game()
for the most part this has worked but when I enter something that isn't number it says "4 is not a number"
ex.
Guess a number between 1 and 10: 3
my number is greater than 3
Guess a number between 1 and 10: water
3 isn't a number!
why doesn't it say "water isn't a number"?
2 Answers
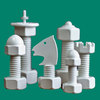
Steven Parker
231,268 PointsThat's because "guess" is assigned using the result of converting the input to a number. If that conversion fails, "guess" still contains the last number that was successfully converted.
To do what you have in mind, you can add another variable to keep the original answer around and use it in message:
try:
# get a number guess from the player
answer =input("Guess a number between 1 and 10: ")
guess = int(answer)
except ValueError:
print("{} isn't a number!".format(answer))
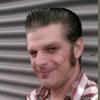
Tobiah Woeltjen
874 PointsIt seems like this is incorrect in the video.
Steven, you answer helped me figure out how to fix this.
Another way to do this would be
try:
# get a guess from the player
guess = input("Guess a number between 1 and 10: ")
guess = int(guess) # just reassign the value
except ValueError:
print("{} is not a number!".format(guess))```
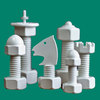
Steven Parker
231,268 PointsThat would prevent the creation of a new variable, but the downside is now "guess" is used for different things at different times. Sometimes it will be a string, and other times a number.
While the program will function the same this way, it can make debugging future issues more complicated and make the program more difficult to read and comprehend by other developers.