Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial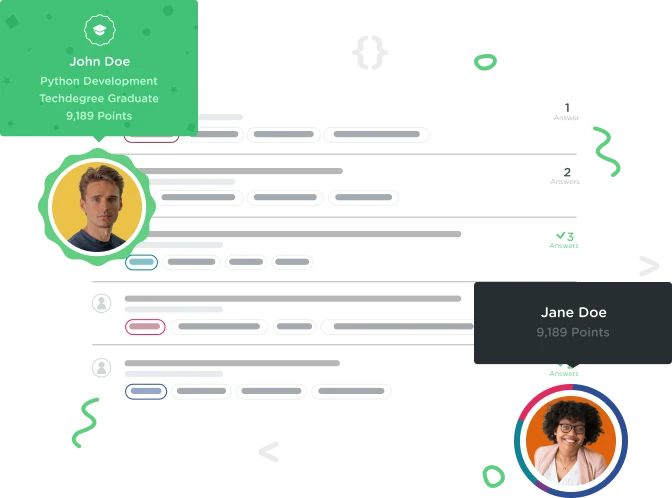

lilysu3
2,518 Pointsnum_teachers challenge: why doesn't this code work?
def num_teachers(teachers_courses): teachers_courses = {} number_of_teachers = len(teachers_courses.keys()) return int(number_of_teachers)
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teachers_courses):
teachers_courses = {}
number_of_teachers = len(teachers_courses.keys())
return int(number_of_teachers)
2 Answers
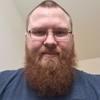
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsAll you have to do is to return the number of teachers, and because there's only 1 teacher in each item you can do it very simple like this
def num_teachers(teachers_courses):
return len(teachers_courses)
# this fails because
def num_teachers(teachers_courses):
teachers_courses = {} # here you set teachers_courses to an empty dict
number_of_teachers = len(teachers_courses.keys()) # getting the length of the empty dict
return int(number_of_teachers) # returning 0 because the dict is empty
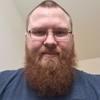
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsI'm not sure about the new code you shared, but since there is 1 teacher pr item in teachers_list you could just return len(teachers_list) like this
def num_teachers(teachers_list):
return len(teachers_list)
Edit: The code might be failing because you print() instead of return
Jordan Pierre
16,601 PointsJordan Pierre
16,601 PointsUPDATE
I figured it out. The only problem was the code was looking for num_teachers and I had num_teacher as the name of the function.
Henrik,
I wrote my code a little differently. It worked in workspaces but not in the code challenge. I would just like to know why it's not working or if the way I wrote it is ok.