Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial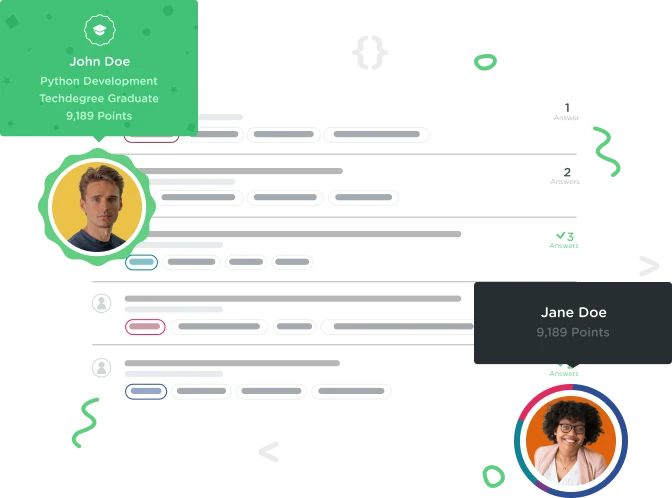
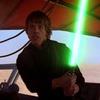
darrian thomas
8,481 PointsObject Challenge part II
Noticed some differences in our code but still the same output. I did not separate my programming from my student objects either but I understand the good organization practice behind doing that. I was just wondering why use a 'student var; ' from the video and a for loop instead of a for in loop?
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
var html = '';
for (student in students) {
html += '<h2>Student: ' + students[student].name + '</h2>';
html += '<p>Track: ' + students[student].track + '</p>';
html += '<p>Points: ' + students[student].points + '</p>';
html += '<p>Achievements: '+ students[student].achievements + '</p>';
}
print(html);
1 Answer
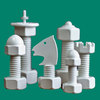
Steven Parker
231,169 PointsThe student var with the conventional for loop allows for more concise property access. But you could get the benefits of both versions by using of instead of in:
for (student of students)
html += '<h2>Student: ' + student.name + '</h2>'
+ '<p>Track: ' + student.track + '</p>'
+ '<p>Points: ' + student.points + '</p>'
+ '<p>Achievements: ' + student.achievements + '</p>';
(I streamlined it a bit further by making the loop just one statement)