Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial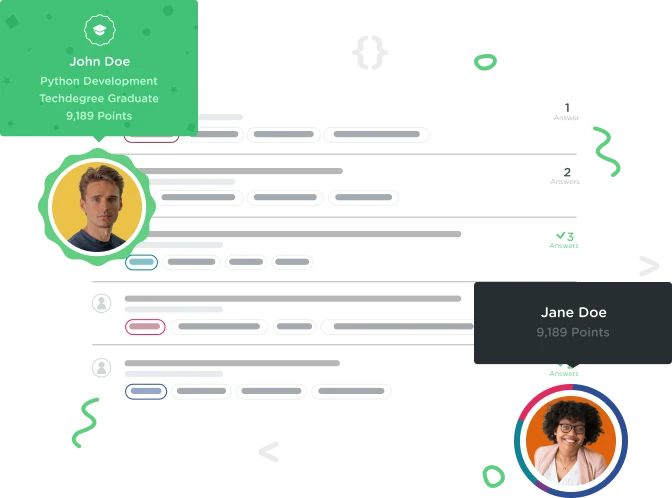
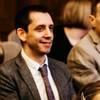
Leon Segal
14,754 PointsObject literal
Could anyone explain in simple terms what the difference is between an object and an object literal?
I have googled it and been on stack overcry or whatever and still can't seem to find a decent answer!
Thanks Leon
5 Answers
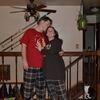
Julie Myers
7,627 PointsAn object is one way of organizing your data in javaScript. It allows you to create once and use many times. For example:
/* Let's say you want to create a way to organize your friends and their car. Instead
of creating a bunch of separate data like so: */
var firstName = "Chloe";
var lastName = "Outlander";
var carBrand = "Honda";
var carModel = "Civic";
function getFullName() {
console.log(firstName + " " + lastName);
}
function getCar() {
console.log(carBrand);
}
// You can organize it into one neat place using objects, like so:
var friends = {
firstName: "Chloe",
lastName: "Outlander",
getFullName() {
console.log(this.firstName + " " + lastName);
}
}
var car = {
carBrand: "Honda",
carModel: "Civic",
getCar: function() {
console.log(this.carBrand);
}
}
An object literal is one of 4 common ways of creating an object. The example above uses object literal notation to create an object. Another way of creating an object is using the constructor function with the new keyword.
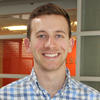
Stephen Gheysens
11,935 PointsHi Leon,
An object literal declaration looks like this:
var obj = {
"property1": "words",
"property2": "more words"
}
and declares an object with specific properties. Due to prototypal inheritance in Javascript, this is technically an instance of the Object "class", just with extra properties.
A "traditional" object declaration follows the patterns from Object-Oriented languages like Java to explicitly declare a new instance of the Object class:
var obj2 = new Obj();
If we wanted this obj2 to behave like the first one, we'd have to add another two lines with:
obj2.property1 = "words";
obj2.property2 = "more words";
It's a subtle difference, but when it comes time to write complicated Javascript applications, you'll be using inheritance a lot more, and adding/removing properties (especially properties that are functions) will be very important.
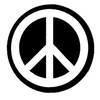
john larson
16,594 Pointssorry I meant:
var object = {
}
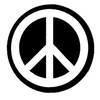
john larson
16,594 PointsJulie, that was beautifully said :D
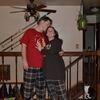
Julie Myers
7,627 PointsThanks, John. :)
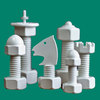
Steven Parker
230,274 PointsStephen is close, but a generic literal object would look more like this:
var obj = {
property1: "words",
property2: number
}
Property names and numeric or boolean values would not have quotes. When everything is quoted, the item in question is probably a JSON object, which is a special case.
The difference between and object and object literal is similar to an ordinary variable and a literal value. In the case of a literal, the value(s) is(are) supplied in the code when the object/variable is defined.
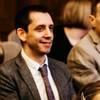
Leon Segal
14,754 PointsThanks for the answers.
Actually I found something useful on wiki:
I can't vouch for the veracity of the article though, as I am still getting to grips with programming.

nathanielcusano
9,808 PointsIn the video it would appear that "dice" is the object literal. Simple as that.
john larson
16,594 Pointsjohn larson
16,594 PointsHaving read the answers I appreciate leon asking for simple explanation. I often find I will get 10 - 50% of an answer. That is, the answer is far above what I can grasp. New topics are brought in leaving me with more questions and still not getting a plain understanding. The closest grasp I thought I had of what an object literal was was this:
But now I don't know.