Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial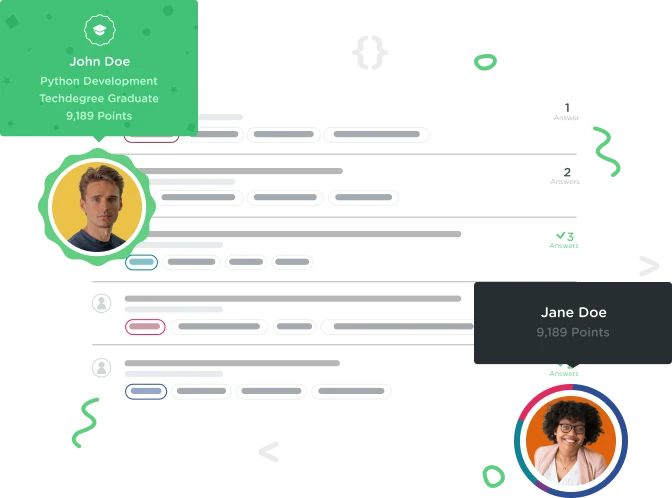

Sam Parkash
6,645 Pointsobject literals vs properties
Hi,
Can someone please explain to me the difference and benefit of using an object literal over a property? From my understanding so far they are the same thing except object literals just let you get away with having a space in your property name or something else that is usually invalid.
3 Answers
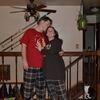
Julie Myers
7,627 PointsAn object literal and a property are two completely different things. An object literal is one way of creating an object, and an object has methods and properties. A property is a part of what makes up an object. For example:
//This is creating an object using object literal technique:
var myObject = {
name: "Alice",
age: 18,
getName: function() {
console.log(this.name);
}
}
/*
name and age are both properties of the object myObject.
getName is a method of the object myObject.
*/
There are other ways of creating an object such as using the constructor function with the new keyword.

Sam Parkash
6,645 PointsThanks :)
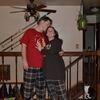
Julie Myers
7,627 PointsYou're welcome. :)
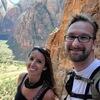
Jeremy Castanza
12,081 PointsSam, I think that your question was just missing some of the correct terminology. However, Julie provided a good explanation for the way that the question was worded.
I think you might have been getting at the difference between dot notation versus square bracket syntax - when it comes to calling a key value pair for a property or calling a method.
Ultimately, dot notation is probably more widely used and preferred
myObject.name
Rather than square bracket syntax...
myObject["name"]
I think what Andrew was getting at is that there's more than one way to get your property information. This could be useful if let's say that I built an object like this and you're trying to access my object for your own program. For example:
var myObject = {
"Aren't I cool?": "Alice"
}
If I built my object this way, you would be forced to change the key for my object. Changing other people's code is always a dicey proposition since you'll have to keep doing it upon updates. Otherwise, you could access the property by using square bracket notation like this:
myObject["Aren't I cool?"]
Hope this helps!
A X
12,842 PointsA X
12,842 PointsSam Parkash Thanks for asking this. I was wondering the same thing.