Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial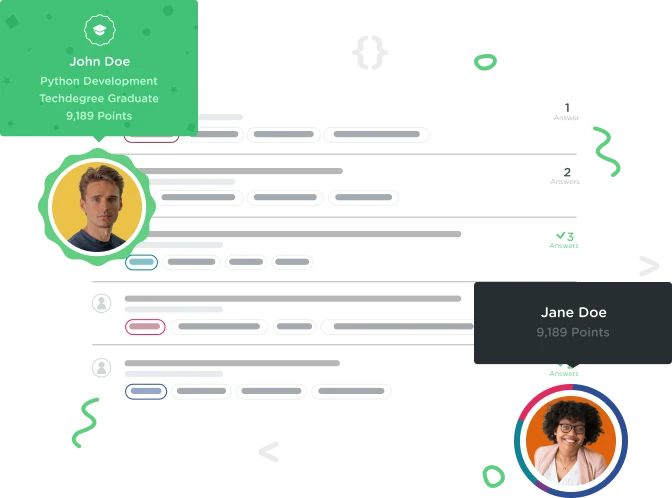

Michael Dean
821 PointsObject Oriented Assignment
I wrote the following, but I don't understand why I get the error (Cannot convert return expression of type '[Person]' to return type 'String') on the return line.
struct Person {
let firstName: String
let lastName: String
}
func fullName(firstName: String, lastName: String) -> String {
var name = [Person]()
let myName = Person(firstName: "Michael", lastName: "Dean")
name.append(myName)
return name
I also don't know how the code the part of the assignment to output the name separated by a space.
2 Answers
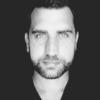
Jhoan Arango
14,575 PointsHello Michael,
To answer your question. You are trying to return an array instead of the "String" you said you were going to return from the method written above.
Remember, when you specify on the signature of the method "fullName" that you will be returning a "String" then that's what the method should return. It will give you an error if you try to return any other type that is not a "String"..
In this particular case you are trying to return an Array, hence the error.
Also, when you write a "Function" inside a type, it is called a "method" or "instance method" since in order for you to use it, you have to create an instance of the type you are defining. In your code, you wrote that outside of the body of the Type and therefore is a "function" or stand alone function. You have no access to it through an instance of your "Person" type.
Let's fix it:
/// Here we have your type
struct Person {
let firstName: String
let lastName: String
/// with-in the body of the type we create the method
/// to return a full name when an instance of this type
/// exist.
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
In the method above, we are using the properties from "Person" and interpolating them to create one full name.
USAGE:
let person = Person(firstName: "Michael", lastName: "Jordan")
print(person.fullName()) // Prints Michael Jordan
Hope this helps

Michael Dean
821 PointsThank you for your response. I'm getting an error on the print line with the following code.
struct Person {
let firstName: String
let lastName: String
}
func fullName(firstName: String, lastName: String) -> String {
return "\(firstName) \(lastName)"
}
let person = Person(firstName: "Michael", lastName: "Jordan")
print(person.fullName())
The error is "Value of type 'Person' has no member 'fullName'".
The first 7 lines of code (through the func statement) were provided in the challenge.
I do have a better understanding of "return" which I didn't have before, Maybe I need to review a previous video on the subject.
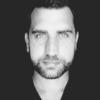
Jhoan Arango
14,575 PointsMichael,
Please observe your opening brackets and closing brackets "{ }". This is very important because that is what defines the body of a type, method, switch etc.
You are defining the method outside of the "Person" type. That's why I explain on the previous answer about "Methods" and "Functions". Right now, how you have it you do not have access to that function. Put it inside the type and you will have access to it when you have an instance of your "Person" type.