Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial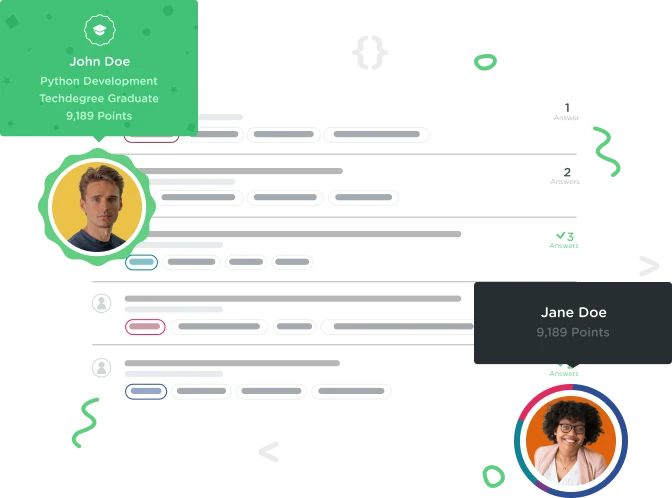

Ary de Oliveira
28,298 PointsObject Oriented Swift 2.0
Challenge Task 1 of 1 Let's get in some practice creating a class. Declare a class named Shape with a variable property named numberOfSides of type Int. Remember that with classes you are required to write an initializer method. Once you have a class definition, create an instance and assign it to a constant named someShape.
Swift no like my code ...
// Enter your code below
class Shape {
var numberOfSides: Int
}
let simeShape = numberOfSides
9 Answers
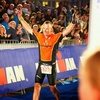
Steve Hunter
57,712 PointsOK - so we have a class called Shape
. It has one property - a whole number (integer) stored in the member variable numberOfSides
. Every instance of Shape
has this property.
When an instance of Shape
is created, a default piece of code runs. In Swift this is termed the initializer, or init
, method. More generally it is called a constructor as it is used to construct the instance of the class.
The init
method here has one parameter passed in; a number. Inside the method, the instance gets its numberOfSides
variable set to that same number. I added an additional name for clarity, I called that sides
as that's shorter to type than numberOfSides
, perhaps! That name can be used when calling the init
method to identify the purpose of the number you are passing in.
So, init
looks like:
init(sides numberOfSides: Int){
self.numberOfSides = numberOfSides
}
That's what runs when the instance is created. To create the instance we decide on a name; the challenge wanted someShape
and then call the class name and pass in the parameters the constructor needs. That looks like:
let someShape = Shape(sides: 3)
Does that make more sense now?
Steve.
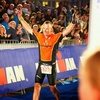
Steve Hunter
57,712 PointsHi Ary,
You need to create the constructor too - the init
method is essential.
Then, the instance is assigned to someShape
:
class Shape{
var numberOfSides: Int
init(sides numberOfSides: Int){
self.numberOfSides = numberOfSides
}
}
let someShape = Shape(sides: 3)
Happy with that?
Steve.

Rich Braymiller
7,119 Pointslet someShape = Shape(sides: 3)
the sides: 3...guess im confused...i know that comes from the init parameter...but...just trying to visualize it in my mind but having some trouble...

Ary de Oliveira
28,298 PointsThanks 2 Good .... Cool...

Rich Braymiller
7,119 Pointsthis kind of confuses me ...
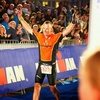
Steve Hunter
57,712 PointsWhich part is causing the problem?
Steve.
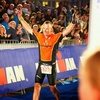
Steve Hunter
57,712 PointsHi Rich,
This comes from the init
method/constructor:
init(sides numberOfSides: Int){
self.numberOfSides = numberOfSides
}
In there, we know that we're going to pass a Int
into the init
method when we create an instance of the Shape
class, right? Are you OK with that part?
Within the init
method the bit of data containing the number is called numberOfSides
so that's what we use inside the method to do things with the value passed in. However, we have also created a separate name, sides
inside the method signature for the init
method. Here: init(sides numberOfSides: Int)
. We can use that outside the class just to make calling the init
method a little clearer, perhaps.
So, rather than calling the method with Shape(numberOfSides: 3)
we can use the, perhaps, clearer, Shape(sides: 3)
. Whilst this isn't hugely clearer in this example, further on in the course, you'll see examples where there are multiple parameters where this name solution really helps add clarity.
Let me know if that's a bit clearer for you, else I'll give it another go!
Steve.

Ary de Oliveira
28,298 PointsThe result is Shape , correct!
Thanks ... All good ... Steve.
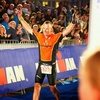
Steve Hunter
57,712 PointsNo problem! :-)

Diana Tiffany Bosso
3,188 PointsIt might be a dumb question but where the 3 comes from?
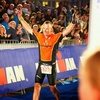
Steve Hunter
57,712 PointsHi Diana,
I can't access the challenge for this as the course is retired. However, either the challenge stipulated to create a Shape
with 3 sides, or the challenge just asked to create an instance of Shape
and on that day, I chose to use the value 3. I really don't remember as I posted back in 2015.
Sorry I can't be more specific.
Steve.

Diana Tiffany Bosso
3,188 Pointsthanks anyway!