Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial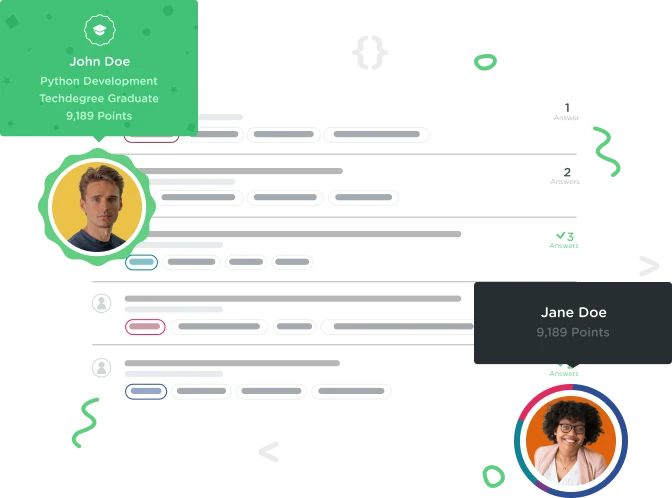
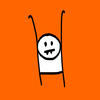
Mike Bailey
823 PointsObject Oriented Swift challenge...
OK - here's the challenge:
--
Given the struct below in the editor, we want to add a method that returns the person’s full name. Declare a method named fullName() that returns a string containing the person’s full name. Note: Make sure to allow for a space between the first and last name
And my code thus far:
struct Person {
let firstName: String
let lastName: String
func fullName(nameOne: String, nameTwo: String) -> [Person]{
var results = [Person]()
let nameOne = "Elvis"
let nameTwo = "Presley"
return results
}
}
I'm told I have to:
"Make sure you're adding an instance method named fullName() to the struct definition"
What am I missing here?
struct Person {
let firstName: String
let lastName: String
func fullName(nameOne: String, nameTwo: String) -> [Person]{
var results = [Person]()
let nameOne = "Elvis"
let nameTwo = "Presley"
return results
}
}
1 Answer
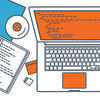
Joshua Nilsson
846 PointsJust do this and It should work! But make sure you mark my answer as the best if that is ok! Thanks! struct Person { let firstName: String let lastName: String
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "John", lastName: "Doe") let myFullName = aPerson.fullName()
Matt Skelton
4,548 PointsMatt Skelton
4,548 PointsImagine we've already created an instance of Person using the following code:
let aPerson = Person(firstName: "Elvis", lastName: "Presley")
By creating an instance of Person in this way, we're using the default initialiser within our struct to assign our firstName and lastName values to our new instance.
Bearing that in mind, let's take a look at fullName function and what we want to achieve.
Declare a method named fullName() that returns a string containing the person’s full name.
At this point, we already have the first and last name values that we need to be assigned to our instance variables, so we don't need to worry about adding them as parameters to our function. We just need to figure out a way in which to combine them into one string that will be returned at the end of the function.
And that should do it! I hope this has cleared things up a little. Good luck!