Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial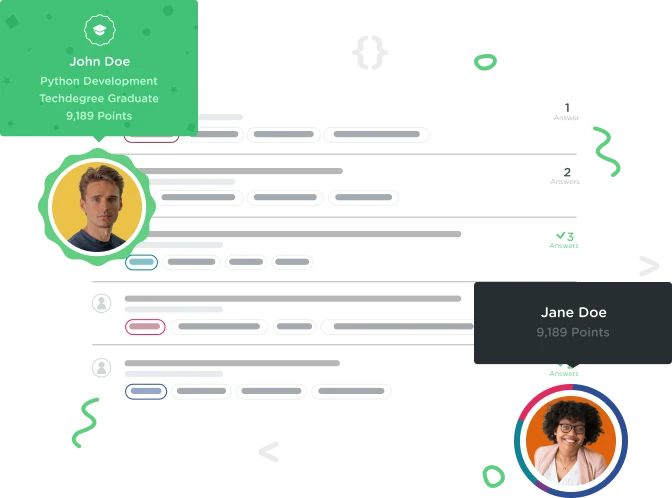

John David
848 PointsObject syntax
Why doesn't the object need a closing semicolon to end the statement before the closing bracket? Also, with the challenge, why does the method work without calling the function as opposed to referencing it(since the return value is the string)? For example, I tried the following as an answer:
var genericGreet = function() {
return "Hello, my name is " + this.name;
}
var andrew = {
name: "Andrew",
greet: genericGreet();
}
var ryan = {
name: "Ryan",
greet: genericGreet();
}
2 Answers

Earl Varona
13,889 PointsIf the code is inside the bracket (or block) and you're at the end before the closing bracket then it's technically the end of that line of code so the semicolon is not necessary (but I still think it's a good habit to apply it).
I'm not sure if I'm understanding your question correctly, but in your code:
greet: genericGreet();
you are calling the function genericGreet instead of assigning it because you are using the () with the function. The correct way is:
greet: genericGreet;
But if you're using it the way you did, the function should only return the string "Hello, my name is " but should not return this.name because it is not a string but an object.method. 'This' refers to its parent object (which is window, or so) and it will look for the method called name of that parent object.
I hope I made sense. Good luck.

Andrei Fecioru
15,059 PointsIf you want to be strict about it, the absolute correct way of doing it is like this: var someFunction = function(){...};
(note the semicolon at the end of the line). This is because you are writing a statement (consisting of a variable assignment), and all statements in JavaScript should end with semicolons. This is in contrast with a pure function definition (which is not a statement) and for which you do not need a semicolon at the end. Example below:
function someFunction() {
...
} // here you do not need semicolon because this is not a statement.
However, many times the JS interpreter is pretty loose (it allows for some deviations) as long as there is no possibility for confusion. In your case, the presence of the closing bracket symbol and the new line is enough to keep the JS interpreter happy.
Note however, that it is always good practice to end your statements with semicolons. Usually in production, JS code gets minified before being deployed on the server, which means that everything gets squished on a single line. What used to be very clear for the JS compiler before minification, suddenly becomes gibberish when code is minified unless all semicolons are consistently placed at the end of each statement.
When dealing with the exercises in this course I assume you are not applying any minification and you can afford to be more lax about these issues.

Earl Varona
13,889 PointsGreat point Andrei
John David
848 PointsJohn David
848 PointsAfter some thinking I believe it is because the objects are technically variable definitions, and the semicolon should come after the closing bracket(since it is a single statement). I'm still unsure of the reason why genericGreet does not need a pair of call parenthesis.