Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial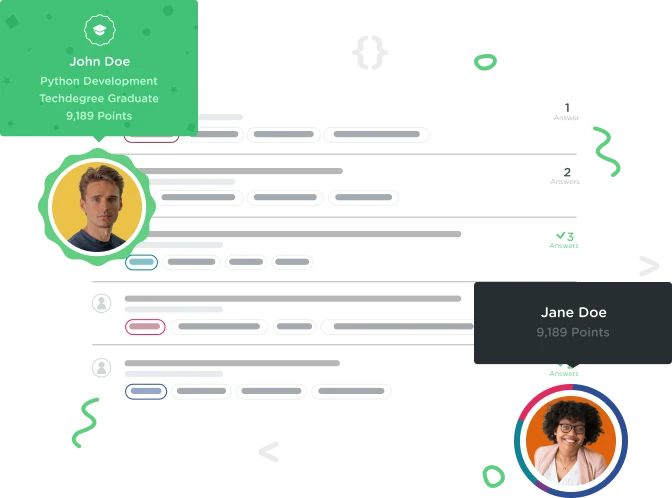

Piers FC
10,402 PointsObject-Orientated Swift 2.0 - Inheritance - last task
I am on the last task of the inheritance section in Swift Object-Orientated Swift 2.0 and I have no idea why my code isn't working.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Docter: Person {
override init(firstName: String, lastName: String){
super.init(firstName: firstName, lastName: lastName)
}
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Docter(firstName: "piers", lastName: "Ebdon")
someDoctor.getFullName
I am getting the error of I need to declare a doctor class, yet I clearly am. It is really irritating that their is no way to see the answer if you are really stuck and can't get help from anyone.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
3 Answers
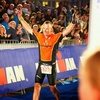
Steve Hunter
57,712 PointsHi Piers,
You don't need to override the init
method - that stays the same.
The only override is the getFullName()
method and you've done that right.
Also, I don't now how picky the compiler is but it wouldn't surprise me if spelling Doctor
wrong has upset it. Maybe correct that too. [EDIT] - Just checked, yes, it wants a class named Doctor
.
Lastly, you call the method with brackets:
someDoctor.getFullName() // brackets added
Let me know how you get on.
Steve.

Piers FC
10,402 PointsHi Steve thanks for helping out.
The final code worked :).
However, I don't fully get why you didn't need to initialize the first part of the class statement. Is it because you inherit the initialisers from the Person class and so if you are not changing them then you do not need to include them?
I thought from the videos that regardless of if you are changing anything or not, you still need to initialise all properties in the superclass. Is that not the case?
Cheers Piers
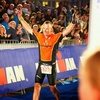
Steve Hunter
57,712 PointsHi Piers,
I believe you are correct, yes. The initializer is inherited if not overridden. An override must include a change; something different must happen. You can, indeed you probably should call the super init if relevant, in such scenarios.
I hope that helps!
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYour final code: