Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial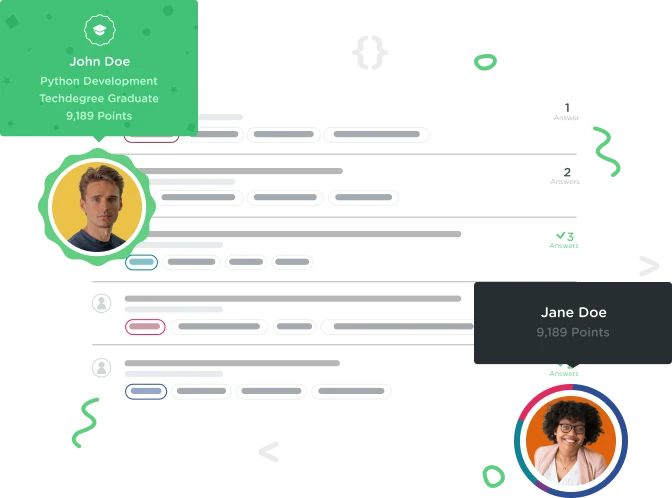

Mary Yang
1,769 PointsObject-Oriented Python: Challenge Task 1 of 1 - Please assist
Hi, I can't for the life of me figure out what is wrong with my code. Can someone please point out where I'm going wrong with this question?
I'm supposed to "Add a score method to Game that takes a player argument that'll be either 1 or 2. Increase that player's value in self.current_score by 1."
As far as I understand, my code does just that, but it's not passing and not giving any errors or hints as to why. Someone please help.
Thanks
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self):
player = input("Would you like to update player 1 or player 2? ")
if player == 1:
self.current_score[0] += 1
elif player == 2:
self.current_score[1] += 1
else:
print("Oops, that's not a 1 or a 2. Please press 1 for player 1 and 2 for player 2")
return self.score
2 Answers
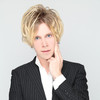
Mikael Enarsson
7,056 PointsThe method is supposed to take an argument for which players score to increase, not have on input(). The argument should come after self.
Also, using if statements in this way is a bit unnecessary in this case, but I like that you want to make sure that the user supplies a valid argument. I would suggest writing it like this though:
if player > 0 or player <= 2:
self.current_score[player - 1] += 1
else:
self.score(input("Oops, that's not a 1 or a 2. Please press 1 for player 1 and 2 for player 2: "))
Also, note that in order to make a function call, you need the opening and closing parentheses:
self.score()
But the error checking isn't really necessary for this challenge.

Chokdee Srisuk
19,384 PointsMaybe you can make score method simple just like below.
def score(self, player): index = player - 1 self.current_score[index] += 1 return self.current_score[index]
Mary Yang
1,769 PointsMary Yang
1,769 PointsOK I see what you did there and how the solution you provided is more elegant.
I just tried my syntax as a solution for the code challenge again but replaced the input with a "player" argument in the function and it passed. I guess my syntax was correct after all, but I needed to provide the argument and not the input. That's what was really driving me crazy last night is I was positive my syntax was correct and couldn't figure out why it was failing.
I like how you replaced four lines of my code with 1 line of yours and it does the same thing. Any suggestions on how to train myself to start thinking in such a way that produces your type of code instead of mine? Does it just come with experience?
Thanks for taking the time to answer my question. This one was really driving me crazy! :)
Mikael Enarsson
7,056 PointsMikael Enarsson
7,056 PointsYeah, you were almost there, I'm glad I could be of help ^^ Although, there was one error in there that should trigger an error, but apparently doesn't, and that is the lack of parentheses after self.score (it should be self.score() for a method call).
It does come with experience, but that's pretty much my advice for how to learn to notice things like that, practice! Write your own code and read through it afterwards to find places were there could be some simplification, read other peoples code and do the same (forums like these are great for this), and, finally, just really think about what you want a line to do. Write out some comments, and see if you can fit it into one line or if you need several. There can also be an issue of flexibility. Maybe in the future you want to use this function to manage 3, 4 or an arbitrary number of players. If you do, you have to rewrite your code for every case.
Those kind of semantic things things are pretty much a matter of preference too. Unless you are working on a project where they require you to follow a certain pattern, just find a way you are comfortable with.
I actually just noticed that I made a mistake too, so that's embarrassing XD
Mary Yang
1,769 PointsMary Yang
1,769 PointsGreat, thanks Mikael. Thanks again for taking the time to provide feedback to my questions. I will definitely put more thought into how long my code is from now on and if it needs to be that long or can be simplified. :D