Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial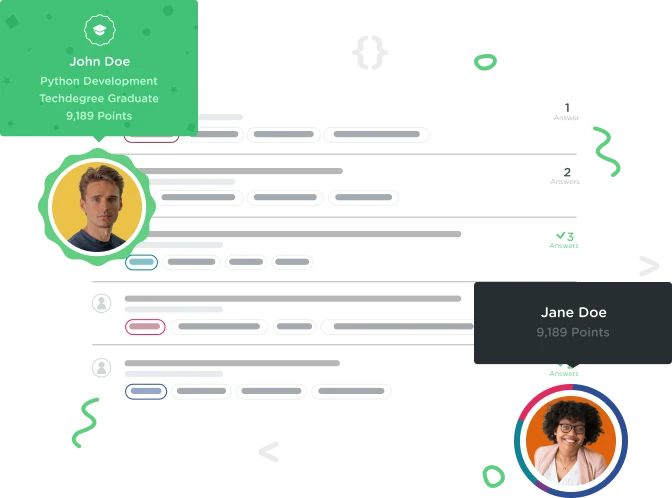

Jacob Carroll
5,957 PointsObject-Oriented Swift Code Challenge: Custom Intializers *Problem*
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) { self.red = red self.green = green self.blue = blue self.alpha = alpha
self.description = (red: "\(red)", green: "\(green)", blue: "\(blue)", alpha: "\(alpha)")
} }
RGBColor.init(red: 86.0, green: 191.0, blue: 131.0, aplha: 1.0)
What is wrong with this code? ( It tells me: Bummer! Don't use the memberwise initializer Swift creates. Add your own custom initializer as specified in the directions. )
I don't know to pass the test. Help?
3 Answers

Alexander Phlippen
947 PointsOkay, so I've got this stage cleared. I don't know why, but the issue did not seem to be the initializer, but that it was not called afterwards.
The following code worked for me:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)";
}
}
let myColor = RGBColor(red: 86.0,green: 191.0, blue: 131.0, alpha: 1.0)
myColor.description

Eric Lakatos
2,338 Pointssince description returns a string, try removing the individual interpolated values and just wrap them all up into a single string
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)";

Alexander Phlippen
947 PointsUPDATE: I've posted a working code snipped in the comment below.
Does not to solve the issue in Swift 3 for me.
My struct looks like this currently:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)";
}
}
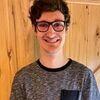
Trent Christofferson
18,846 Pointsit is because you are using strings in the values and the type is set to Double. try:
self.description = (red: red, green: green, blue: blue, alpha: alpha)

Alexander Phlippen
947 PointsUPDATE: I've posted a working code snipped in the comment below.
Also tried this version, wich sadly raises the same error
Bummer! Don't use the memberwise initializer Swift creates. Add your own custom initializer as specified in the directions
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = (red: red, green: green, blue: blue, alpha: alpha)
}
}
Alexander Phlippen
947 PointsAlexander Phlippen
947 PointsPlease see my working code in a comment below. Best, Oliver