Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial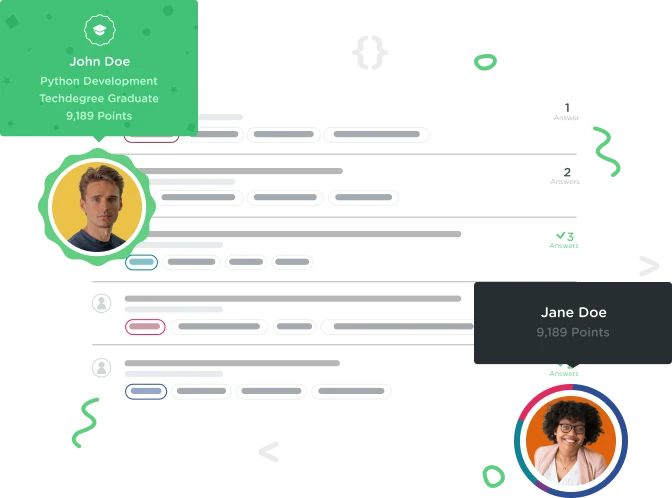
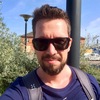
John Romby Andersson
8,453 PointsObjects and Classes Extras - My solution
Hello everyone, I have been very active here at Treehouse learning everything from HTML, CSS, JS and now i´m in the middle of learning Ruby. :-)
So far so good, but I have been neglecting to post anything on the forums thus far. I am however planning on changing that now, since I have finally come to the conclusion that the best way to keep all the new skills learned is to talk about them and of course practice like crazy. ;-)
So anyways, please allow me to put up my code here for the Extra Assignment given at the end of the Ruby Foundations - Stage 2
=begin
This is my first real Ruby App.
I am starting super simple here - The current exercise is giving by Jason Seifer @Treehouse. :)
Here is the assigment info he gave at the end of Objects, Classes, and Variables.
Extra Credit
Input and Printing
Write a small Ruby program that asks you what your name is. Have the program print out the number of characters in your name.
Also have the program print out a message if your name is longer than 25 characters.
=end
class YourName
def ask_name
print "\n\tPlease tell us your name: "
@myname = gets.chomp
puts "\n\n\tHello #{@myname.split[0]} and welcome to this simple Ruby App :)"
end
def name_length
puts "\n\tWould you like to know how many characters there is in your name?"
myname_length = @myname.gsub(/[ ]/,'').length
while true do
print "\n\tPlease type: (Y)es or (N)o: "
myname_ask = gets.chomp
case myname_ask.downcase
when "y", "yes"
myname_comment = "\n\n\tYour name (#{@myname}) is #{myname_length} characters long - and might I add, "
if myname_length > 25
puts myname_comment + "you have one very long name, wow. :D"
else
puts myname_comment + "you have a beautiful name. :)"
end
break
when "n", "no"
puts "\n\n\tOkay, no worries we keep that a secret between us then. ;)"
break
else
puts "\n\n\tSorry I do not know what you want with that answer, please try again. :("
end
end
puts ""
end
end
your_name = YourName.new
your_name.ask_name
your_name.name_length
Please let me know if the code is clean enough or if there is a better way of going about doing it.
Thank you. :-)
Regards, John Romby Andersson
5 Answers
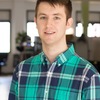
Ethan Lowry
Courses Plus Student 7,323 PointsYour code seems fine generally. There's probably no need for myname_length and myname_ask to be instance variables though - you only use them within that single method so they could just be local variables without the '@'.
Also, while I know they're sometimes helpful, most people would frown on using the START/END SECTION comments in real world Ruby code - after all, the main goal of Ruby is to be as highly readable as possible, so they generally aren't as necessary as they might be in other languages. Your indentation should always make it clear what block of code an 'end' belongs to.
Also I might extract the 25 character check out into its own separate method - Ruby tends to lean towards lots of very small methods that might only contain a couple of lines of code.
And, if you were to do that, you could change your large method to simply return those strings (by leaving off the 'puts') and calling the method like this instead:
def name_length
puts "\n Would you like to know how many characters there is in your name?"
@myname_length = @myname.length
while true do
print "\n Please type: (Y)es or (N)o: "
@myname_ask = gets.chomp
case @myname_ask.downcase
when "y", "yes"
"\n Your name is #{@myname_length}, characters long (including spaces), and might I add, you have a beautiful name :)"
when "n", "no"
"\n Okay, no worries we keep that a secret between us then ;)"
else
"\n Sorry I do not know what you want with that answer :("
end
end
end
def long_name_check
if @myname_length > 25
"\n Hope you don't mind me saying, but that is one super long name you have there :o"
end
end
puts your_name.name_length
puts your_name.long_name_check

Thillai Arasu Narayanan
Full Stack JavaScript Techdegree Student 901 PointsWe can do like this too
class Myname def initialize() puts "Whats your name ?" @name = gets.chomp end
def name_length if @name.length > 25 puts "Your name #{@name} is too long to print" else puts "Your name #{@name} is perfect to print" end end end
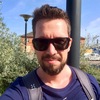
John Romby Andersson
8,453 PointsThank you Ethan, some good points there mate. Changed the variables and removed the 'bad ruby habit' comments in my OP ;)
Will implement the good practice of keep small clean methods later and in my next projects. :)
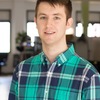
Ethan Lowry
Courses Plus Student 7,323 PointsNo problem man - just remember to leave comments on other people's answers instead of creating a new answer in future ;)
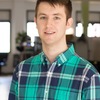
Ethan Lowry
Courses Plus Student 7,323 PointsOh, and remember to select a Best Answer in addition to upvoting whenever you're satisfied with any answer.
Edit: LOL, just made the same mistake as you myself. D'oh.
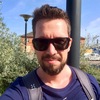
John Romby Andersson
8,453 PointsHaha, you are not gonna get a best answer for that one, but the other one is sure getting one ;)
Cheers.
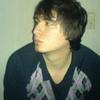
gusf
11,070 PointsMy code:
class YourName
def initialize(name)
puts "Whats your name ?"
@name = gets.chomp
end
def name_length
if @name.length > 25
puts "Your name has #{@name.length} characters and therefore it won't print"
else
puts "Your name #{@name} has #{@name.length} characters."
end end
end
your_name = YourName.new("")
your_name.name_length